tf2 加载未知结构的tfrecord 代码
时间: 2024-06-11 10:04:17 浏览: 108
由于TFRecord可以存储任意的二进制数据,因此如果我们不知道TFRecord的具体结构,那么我们需要进行一些探索和试错。
下面是一些可能有用的代码片段,可以帮助加载未知结构的TFRecord:
```python
import tensorflow as tf
def read_tfrecord(filename):
record_iterator = tf.compat.v1.python_io.tf_record_iterator(path=filename)
for string_record in record_iterator:
example = tf.train.Example()
example.ParseFromString(string_record)
# 打印TFRecord的所有字段和值
for key in example.features.feature:
value = example.features.feature[key].bytes_list.value
print(f"{key}: {value}")
```
上述代码片段可以读取TFRecord文件中的所有记录,并将它们解析为`tf.train.Example`对象。然后,我们可以使用`example.features.feature`属性来访问每个字段的值,并将其打印出来。
如果我们知道TFRecord的预期结构,那么我们可以使用`tf.parse_single_example`函数来解析每个记录。例如,假设我们知道TFRecord包含一个名为`image`的图像字段和一个名为`label`的标签字段,我们可以使用以下代码:
```python
def read_tfrecord(filename):
record_iterator = tf.compat.v1.python_io.tf_record_iterator(path=filename)
for string_record in record_iterator:
example = tf.train.Example()
example.ParseFromString(string_record)
# 解析图像和标签字段
features = {
'image': tf.io.FixedLenFeature([], tf.string),
'label': tf.io.FixedLenFeature([], tf.int64)
}
parsed = tf.io.parse_single_example(string_record, features)
# 将图像解码为张量
image = tf.io.decode_jpeg(parsed['image'])
label = parsed['label']
# 进行一些操作,例如将图像和标签送入模型进行推理
...
```
上述代码片段使用`tf.io.parse_single_example`函数解析图像和标签字段,并将图像解码为张量。然后,我们可以将图像和标签送入模型进行推理。
总之,加载未知结构的TFRecord需要一些探索和试错,但是在解析每个记录时使用`tf.parse_single_example`函数可以大大简化代码。
相关推荐
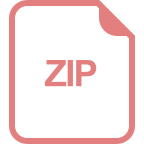
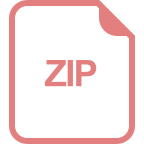














