java编写一个负载均衡中的权重分配算法
时间: 2024-05-14 08:14:02 浏览: 71
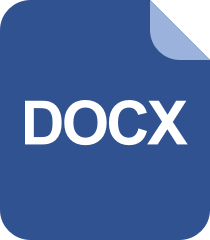
Java负载均衡
以下是一个简单的Java代码示例,用于实现加权轮询(Weighted Round Robin)算法:
```
import java.util.ArrayList;
import java.util.List;
public class WeightedRoundRobin {
private List<Server> serverList;
private int currentIndex = -1;
private int currentWeight = 0;
private int maxWeight = 0;
private int gcdWeight = 0;
public WeightedRoundRobin(List<Server> servers) {
this.serverList = servers;
for (Server server : serverList) {
maxWeight = Math.max(maxWeight, server.weight);
}
gcdWeight = gcdWeight();
}
public Server nextServer() {
while (true) {
currentIndex = (currentIndex + 1) % serverList.size();
if (currentIndex == 0) {
currentWeight -= gcdWeight;
if (currentWeight <= 0) {
currentWeight = maxWeight;
if (currentWeight == 0) {
return null;
}
}
}
if (serverList.get(currentIndex).weight >= currentWeight) {
return serverList.get(currentIndex);
}
}
}
private int gcdWeight() {
int weight = 0;
for (Server server : serverList) {
weight = gcd(weight, server.weight);
}
return weight;
}
private int gcd(int a, int b) {
if (b == 0) {
return a;
} else {
return gcd(b, a % b);
}
}
public static void main(String[] args) {
List<Server> serverList = new ArrayList<>();
serverList.add(new Server("Server1", 4));
serverList.add(new Server("Server2", 2));
serverList.add(new Server("Server3", 1));
WeightedRoundRobin lb = new WeightedRoundRobin(serverList);
for (int i = 0; i < 10; i++) {
Server server = lb.nextServer();
System.out.println("Request " + (i + 1) + " routed to " + server.name);
}
}
}
class Server {
public String name;
public int weight;
public Server(String name, int weight) {
this.name = name;
this.weight = weight;
}
}
```
在此代码示例中,我们首先定义了一个Server类,该类包含服务器的名称和权重。然后我们定义了WeightedRoundRobin类,其中包含服务器列表,当前索引,当前权重,最大权重和最大公约数权重等变量。我们也实现了nextServer()方法,该方法使用加权轮询算法来选择下一个服务器。我们还实现了gcdWeight()和gcd()方法,这些方法用于计算服务器权重的最大公约数。最后,我们在main()方法中创建了一个包含三个服务器的列表,并使用WeightedRoundRobin类来路由请求。
阅读全文
相关推荐
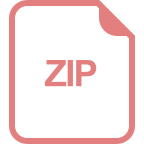
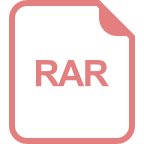
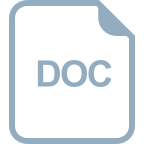
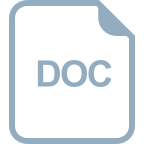
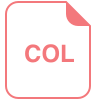
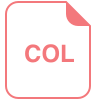
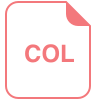
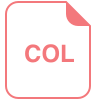
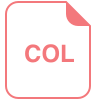
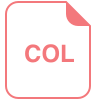
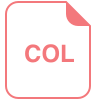
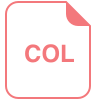
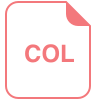
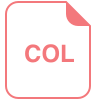
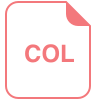
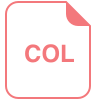
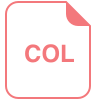
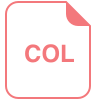