c++调用python接口
时间: 2023-10-09 19:13:29 浏览: 172
C++ 调用 Python 接口的方式主要有两种:通过 Python C API 和通过 Boost.Python 库。
1. Python C API
Python C API 是 Python 官方提供的 C/C++ 调用 Python 接口。使用 Python C API 需要了解 Python 内部的数据结构和函数调用方式。
具体可以参考 Python 官方文档:https://docs.python.org/3/extending/index.html
2. Boost.Python
Boost.Python 是一个开源的 C++ 库,提供了方便的接口,使得 C++ 可以直接调用 Python。
具体可以参考 Boost.Python 的官方文档:https://www.boost.org/doc/libs/1_72_0/libs/python/doc/html/index.html
相关问题
c++调用python接口,传入参数
在C++中调用Python接口通常需要借助于一些库,如Boost.Python、Pybind11或者是第三方的C++绑定工具如SWIG。这些工具允许你在C++中封装Python函数,并提供给Python语言调用。
例如,如果你使用Pybind11,首先你需要在C++代码中声明Python函数:
```cpp
#include <pybind11/pybind11.h>
namespace py = pybind11;
void say_hello(const std::string& name) {
py::gil_scoped_acquire acquire; // 锁住全局解释器锁
py::print("Hello, " + name);
}
PYBIND11_MODULE(example, m) { // 创建模块
m.def("hello", &say_hello); // 绑定函数到module上
}
```
然后,在Python端你可以像这样调用这个函数:
```python
import example
example.hello('World') # 输出 "Hello, World"
```
当你传递参数给`say_hello`时,实际上是将C++的参数转换为Python的对象。如果参数类型可以匹配,转换通常是透明的。
利用Boost::Python实现C++调用python接口
Boost::Python 是一个用于将 C++ 代码与 Python 交互的库。它提供了一个简单的界面来创建 Python 模块并在 C++ 中访问 Python 对象和方法。下面是一个简单的示例代码,展示了如何使用 Boost::Python 实现 C++ 调用 Python 接口:
首先,在 C++ 中需要包含 Boost::Python 库的头文件:
```
#include <boost/python.hpp>
```
然后,创建一个 Python 模块:
```
BOOST_PYTHON_MODULE(my_module) {
// 在这里注册 Python 函数
}
```
接下来,可以在模块中注册 Python 函数:
```
BOOST_PYTHON_MODULE(my_module) {
using namespace boost::python;
def("my_function", my_function);
}
```
其中,my_function 是一个 Python 函数,可以通过以下方式在 Python 中定义:
```
def my_function(args):
# Python 代码
```
最后,在 C++ 中使用 Boost::Python 调用 Python 函数:
```
#include <boost/python.hpp>
using namespace boost::python;
int main() {
// 初始化 Python 解析器
Py_Initialize();
// 导入 Python 模块
object module = import("my_module");
// 调用 Python 函数
object result = module.attr("my_function")(args);
// 将 Python 返回值转换为 C++ 类型
int value = extract<int>(result);
// 释放 Python 解析器
Py_Finalize();
return 0;
}
```
以上是一个简单的 Boost::Python 调用 Python 接口的示例。在实际应用中,还需要处理异常、管理 Python 对象生命周期等问题。
阅读全文
相关推荐






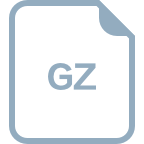



