java List<Entity> 找到Entity中最大的id
时间: 2024-03-11 10:50:26 浏览: 86
可以使用以下代码来实现:
```java
List<Entity> entityList = new ArrayList<>(); // 假设已有实体列表
int maxId = Integer.MIN_VALUE; // 初始化最大id为最小整数值
for (Entity entity : entityList) {
if (entity.getId() > maxId) {
maxId = entity.getId();
}
}
System.out.println("最大id为:" + maxId);
```
其中,`getId()` 是 `Entity` 类中获取 id 的方法,根据实际情况进行调整。这段代码会遍历列表中的每个实体对象,比较其 id 值是否大于当前最大值,如果是则更新最大值。最后输出最大值即可。
相关问题
java尝试将 Map<String,Map<String,LIst<Entity>>> list 转换为普通的 List<entity> 结构,给出效率最优代码,并给出适当注释
可以使用 Java 8 的 Stream API 来进行转换。
先将 Map<String, Map<String, List<Entity>>> 转换为 List<List<List<Entity>>> 结构,然后使用 flatMap 将其扁平化为 List<List<Entity>>,最后再使用 flatMap 将其再次扁平化为 List<Entity>。
以下是代码:
```
List<Entity> result = map.values().stream() // 获取 Map<String, Map<String, List<Entity>>> 的值集合
.map(innerMap -> innerMap.values().stream() // 将 Map<String,LIst<Entity>> 转换为 List<List<Entity>> 结构
.flatMap(Collection::stream)
.collect(Collectors.toList()))
.flatMap(Collection::stream) // 将 List<List<Entity>> 扁平化为 List<Entity>
.collect(Collectors.toList());
```
代码注释如下:
```
List<Entity> result = map.values().stream() // 获取 Map<String, Map<String, List<Entity>>> 的值集合
.map(innerMap -> innerMap.values().stream() // 将 Map<String,LIst<Entity>> 转换为 List<List<Entity>> 结构
.flatMap(Collection::stream) // 将 List<Entity> 扁平化为一个流
.collect(Collectors.toList())) // 将流转换为 List<List<Entity>> 结构
.flatMap(Collection::stream) // 将 List<List<Entity>> 扁平化为 List<Entity>
.collect(Collectors.toList()); // 将扁平化后的集合转换为 List<Entity> 结构
```
这样的代码效率较高,并且可以处理任意深度的嵌套结构。
springboot 将List<Entity>存如redis,怎么拿出来时也是List<Entity>的数据类型
Spring Boot结合Redis存储`List<Entity>`数据时,通常的做法是序列化和反序列化操作。由于Redis是一个键值对存储系统,不直接支持复杂数据类型的持久化,所以我们需要将Java对象转换成可存储的字符串或字节数组形式。以下是步骤:
1. **序列化**:
使用Spring提供的`Jackson`或`Gson`库将`List<Entity>`序列化为JSON字符串或二进制字节流。示例:
```java
ObjectMapper mapper = new ObjectMapper();
String serializedList = mapper.writeValueAsString(list);
```
2. **存储**:
将序列化的数据作为Redis的value,key可以是你选择的一个标识:
```java
String key = "myEntities";
StringSetOperations<String> setOps = redisTemplate.opsForValue();
setOps.set(key, serializedList);
```
或者如果使用的是JedisTemplate,可以考虑使用`opsForHash`来保存整个键值对,键是集合名,值是序列化的字符串。
3. **反序列化**:
当你需要从Redis取出数据时,先从Redis获取value,然后解序列化回`List<Entity>`:
```java
@Autowired
private RedisTemplate<String, Object> redisTemplate;
List<Entity> deserializedList;
try {
deserializedList = mapper.readValue(redisTemplate.opsForValue().get(key), new TypeReference<List<Entity>>() {});
} catch (JsonProcessingException e) {
// 处理异常
}
```
注意:这里假设你的Entity实现了`Serializable`接口。如果不是,你需要提供一个自定义的序列化策略,例如`JacksonObjectMapperConfigurer`或`GsonTypeAdapter`。
阅读全文
相关推荐
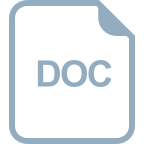
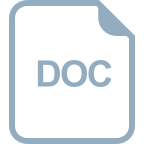
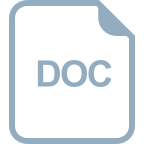













