假设机器人系统通过RS485与某电机控制器通信,采用MODBUS RTU协议。电机控制器内部存放电机电流的起始地址是0x1234(每个地址存在1个字节),电流值占用4个地址(4字节整数,以0.1A为单位,大端格式)。利用功能码3读取该电机控制器内部保存的电机当前电流值。用C语言编写发送03命令和接收数据并处理得到电流值的程序。(不包括通信部分程序)
时间: 2024-03-19 15:45:36 浏览: 87
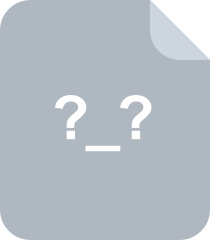
一个ModBus RTU程序(支持01、02、03、05、06、15、16功能码).c
下面是一个简单的C语言程序,用于发送03命令和接收数据并处理得到电流值:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define DEVICE "/dev/ttyUSB0"
#define BAUDRATE B115200
int main()
{
int fd = 0;
struct termios options;
int res = 0;
// 打开串口
fd = open(DEVICE, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1)
{
perror("open");
return -1;
}
// 配置串口
fcntl(fd, F_SETFL, FNDELAY);
tcgetattr(fd, &options);
cfsetispeed(&options, BAUDRATE);
cfsetospeed(&options, BAUDRATE);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cc[VTIME] = 0;
options.c_cc[VMIN] = 1;
tcsetattr(fd, TCSANOW, &options);
// 发送03命令
unsigned char buf[8] = {0x01, 0x03, 0x12, 0x34, 0x00, 0x04, 0x84, 0x0a};
res = write(fd, buf, 8);
if (res == -1)
{
perror("write");
return -1;
}
// 读取数据
unsigned char recv_buf[256] = {0};
res = read(fd, recv_buf, 256);
if (res == -1)
{
perror("read");
return -1;
}
// 处理数据
unsigned char data_buf[4] = {0};
memcpy(data_buf, recv_buf + 3, 4);
int current = (data_buf[0] << 24) | (data_buf[1] << 16) | (data_buf[2] << 8) | data_buf[3];
printf("Current: %d.%dA\n", current / 10, current % 10);
close(fd);
return 0;
}
```
注意,此程序中使用的是Linux系统下的串口通信库,Windows系统下的库可能有所不同。此外,具体的设备文件名(如`/dev/ttyUSB0`)和波特率(如`B115200`)需要根据实际情况进行修改。
阅读全文
相关推荐
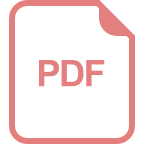
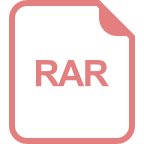
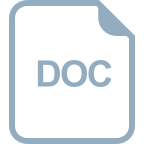
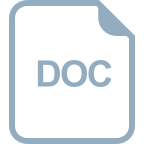
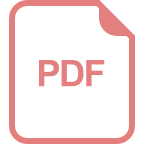
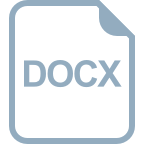
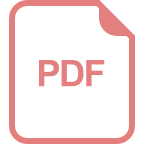
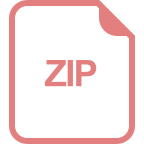
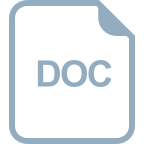
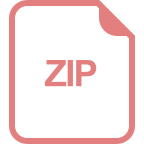
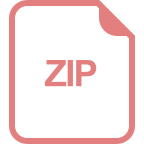
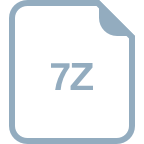
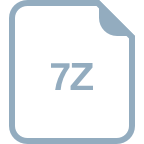
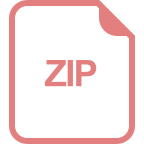
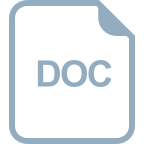
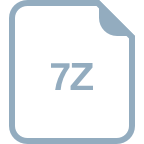
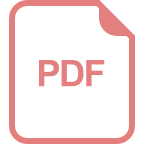