(3)使用pandas、numpy、matplotlib实现每个种类鸢尾花的Sepal.Length折线图 (4)使用pandas、numpy、matplotlib实现每个种类鸢尾花的Sepal.Width折线图 (5)使用pandas、numpy、matplotlib实现每个种类鸢尾花的Petal.Length柱状图 (6)使用pandas、numpy、matplotlib实现每个种类鸢尾花的Petal.Width柱状图 注:导入鸢尾花数据后的DataF
时间: 2023-11-15 17:06:28 浏览: 182
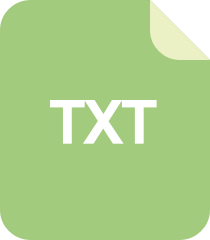
iris文本数据集,鸢尾花分类,用于numpy练习
rame对象为df,其中包含四列分别为Sepal.Length, Sepal.Width, Petal.Length和Petal.Width,以及一列Species表示鸢尾花的种类(Iris-setosa, Iris-versicolor, Iris-virginica)。以下是实现这些图形的代码:
```
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# 导入鸢尾花数据
df = pd.read_csv('iris.csv')
# 生成每个种类鸢尾花的Sepal.Length折线图
fig, ax = plt.subplots()
for species in df['Species'].unique():
x = df[df['Species']==species]['Sepal.Length']
y = np.arange(len(x))
ax.plot(y, x, label=species)
ax.set_xticks(np.arange(len(x)))
ax.set_xticklabels(y)
ax.set_xlabel('Index')
ax.set_ylabel('Sepal.Length')
ax.legend()
plt.show()
# 生成每个种类鸢尾花的Sepal.Width折线图
fig, ax = plt.subplots()
for species in df['Species'].unique():
x = df[df['Species']==species]['Sepal.Width']
y = np.arange(len(x))
ax.plot(y, x, label=species)
ax.set_xticks(np.arange(len(x)))
ax.set_xticklabels(y)
ax.set_xlabel('Index')
ax.set_ylabel('Sepal.Width')
ax.legend()
plt.show()
# 生成每个种类鸢尾花的Petal.Length柱状图
fig, ax = plt.subplots()
for i, species in enumerate(df['Species'].unique()):
x = df[df['Species']==species]['Petal.Length']
ax.bar(i, x.mean(), yerr=x.std(), label=species)
ax.set_xticks(np.arange(len(df['Species'].unique())))
ax.set_xticklabels(df['Species'].unique())
ax.set_xlabel('Species')
ax.set_ylabel('Petal.Length')
ax.legend()
plt.show()
# 生成每个种类鸢尾花的Petal.Width柱状图
fig, ax = plt.subplots()
for i, species in enumerate(df['Species'].unique()):
x = df[df['Species']==species]['Petal.Width']
ax.bar(i, x.mean(), yerr=x.std(), label=species)
ax.set_xticks(np.arange(len(df['Species'].unique())))
ax.set_xticklabels(df['Species'].unique())
ax.set_xlabel('Species')
ax.set_ylabel('Petal.Width')
ax.legend()
plt.show()
```
这段代码会生成四个图形,分别为每个种类鸢尾花的Sepal.Length折线图,Sepal.Width折线图,Petal.Length柱状图和Petal.Width柱状图。每个图形都包含了三个种类的鸢尾花的数据,并且使用了合适的标签和图例来区分不同的种类。
阅读全文
相关推荐
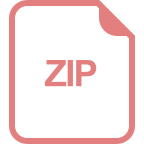


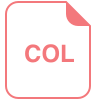
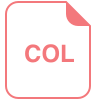
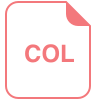
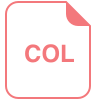
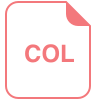
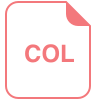
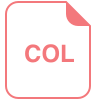
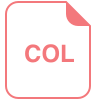
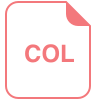
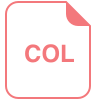
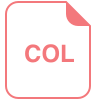
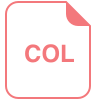
