matlab 开发俄罗斯方块
时间: 2023-10-27 10:03:17 浏览: 76
Matlab是一种强大的编程语言和开发环境,可以用来开发各种各样的应用程序,包括俄罗斯方块游戏。
首先,我们需要创建一个图形用户界面(GUI)来显示游戏的界面和交互。可以使用Matlab的GUI设计工具或编程来实现。在界面中,我们可以添加一个方块矩阵来表示游戏的主区域,以及其他元素,如下一个方块预览、分数显示等。
接下来,我们需要编写代码来实现游戏的逻辑。首先,我们需要定义一个方块类来表示游戏中的方块,其中包含方块的形状信息、旋转状态和位置信息。然后,我们可以使用随机函数生成下一个要落下的方块,并根据用户的输入(如键盘按键)来控制方块的移动、旋转和下落。
为了实现方块的移动和碰撞检测,我们可以使用Matlab提供的数组操作和条件判断语句。例如,可以使用移动和旋转功能将方块的位置和形状信息更新,然后通过与已落下的方块进行碰撞检测来确定是否可以进行移动或旋转操作。
在游戏进行中,当一行方块填满时,我们需要将该行方块清除,并更新分数。我们可以使用Matlab的循环和条件判断语句来实现这个功能。
最后,我们需要添加游戏结束的条件和相应的提示信息。当新生成的方块无法再进行移动时,游戏结束,可以通过Matlab的弹窗或在界面上显示相应的信息来提示玩家。
综上所述,通过使用Matlab的图形用户界面设计工具和编程语言,我们可以开发一个简单而又有趣的俄罗斯方块游戏。该游戏具有方块的生成、移动和旋转功能,碰撞检测和消除行方块的功能,以及游戏结束的判断和提示功能。
相关问题
matlab实现俄罗斯方块
可以使用MATLAB来实现俄罗斯方块游戏。下面是一个简单的示例代码,帮助你开始:
```matlab
function Tetris()
% 游戏设置
rows = 20; % 游戏区域行数
columns = 10; % 游戏区域列数
% 创建游戏区域
gameArea = zeros(rows, columns);
% 创建一个新的方块
currentPiece = createPiece();
% 游戏循环
while true
% 显示游戏区域和当前方块
drawGameArea(gameArea, currentPiece);
% 检查用户输入
userInput = input('请输入方向 (a: 左移, d: 右移, s: 下移): ', 's');
% 移动方块
switch userInput
case 'a'
currentPiece.x = currentPiece.x - 1;
case 'd'
currentPiece.x = currentPiece.x + 1;
case 's'
currentPiece.y = currentPiece.y + 1;
end
% 检查碰撞和边界
if checkCollision(gameArea, currentPiece) || checkBoundary(currentPiece, rows, columns)
% 方块碰撞或超出边界,将方块固定在游戏区域中
gameArea = fixPiece(gameArea, currentPiece);
% 检查是否有一行已满
fullRows = findFullRows(gameArea);
if ~isempty(fullRows)
% 移除已满的行
gameArea(fullRows, :) = [];
% 在顶部添加新的空行
gameArea = [zeros(length(fullRows), columns); gameArea];
end
% 创建一个新的方块
currentPiece = createPiece();
end
end
end
function piece = createPiece()
% 定义所有可能的方块形状
shapes = [1 1 1 1;
1 1 1 0;
1 1 0 0;
1 1 0 0];
% 随机选择一个方块形状
shapeIndex = randi(size(shapes, 1));
% 创建方块对象
piece.shape = shapes(shapeIndex, :);
piece.x = ceil(size(shapes, 2) / 2);
piece.y = 1;
end
function drawGameArea(gameArea, currentPiece)
% 绘制游戏区域
for i = 1:size(gameArea, 1)
for j = 1:size(gameArea, 2)
if gameArea(i, j)
fprintf('* ');
else
fprintf(' ');
end
end
fprintf('\n');
end
% 绘制当前方块
for i = currentPiece.y:currentPiece.y + size(currentPiece.shape, 1) - 1
for j = currentPiece.x:currentPiece.x + size(currentPiece.shape, 2) - 1
if currentPiece.shape(i - currentPiece.y + 1, j - currentPiece.x + 1)
fprintf('* ');
else
fprintf(' ');
end
end
fprintf('\n');
end
end
function collision = checkCollision(gameArea, currentPiece)
% 检查方块在游戏区域中是否与已有方块发生碰撞
collision = false;
for i = currentPiece.y:currentPiece.y + size(currentPiece.shape, 1) - 1
for j = currentPiece.x:currentPiece.x + size(currentPiece.shape, 2) - 1
if currentPiece.shape(i - currentPiece.y + 1, j - currentPiece.x + 1) && gameArea(i, j)
collision = true;
return;
end
end
end
end
function boundary = checkBoundary(currentPiece, rows, columns)
% 检查方块是否超出游戏区域边界
boundary = false;
if currentPiece.x < 1 || currentPiece.x + size(currentPiece.shape, 2) - 1 > columns || currentPiece.y + size(currentPiece.shape, 1) - 1 > rows
boundary = true;
end
end
function gameArea = fixPiece(gameArea, currentPiece)
% 将方块固定在游戏区域中
for i = currentPiece.y:currentPiece.y + size(currentPiece.shape, 1) - 1
for j = currentPiece.x:currentPiece.x + size(currentPiece.shape, 2) - 1
if currentPiece.shape(i - currentPiece.y + 1, j - currentPiece.x + 1)
gameArea(i, j) = 1;
end
end
end
end
function fullRows = findFullRows(gameArea)
% 找到已满的行
fullRows = [];
for i = 1:size(gameArea, 1)
if all(gameArea(i, :))
fullRows(end + 1) = i;
end
end
end
```
这是一个简化的实现,你可以根据需要进行修改和扩展。运行函数 `Tetris()` 即可开始游戏。使用键盘上的方向键来控制方块的移动。
请注意,MATLAB并不是一个专门用于游戏开发的语言,因此这只是一个简单的示例。如果你需要更复杂的游戏,可能需要使用其他编程语言和游戏引擎来实现。
使用matlab创建俄罗斯方块
很高兴可以回答您的问题。关于使用Matlab创建俄罗斯方块的问题,我可以为您提供一些信息。Matlab是一个强大的科学计算软件,它可以用于许多不同的应用程序,包括游戏开发。您可以利用Matlab的图形界面工具箱和编程功能来构建俄罗斯方块游戏。如果您对Matlab编程并不熟悉,我可以为您推荐一些在线课程或教程,以帮助您更好地了解如何使用该软件来创建您的游戏。希望我的回答能够对您有所帮助!
相关推荐
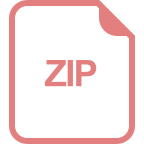












