用java的swing做一个界面,界面可以新建商品列表,列表要求有自定义字体和图标,并且鼠标滚轮可以控制下滑,隐藏滚动条
时间: 2024-04-29 13:23:49 浏览: 102
以下是一个简单的Java Swing界面,实现了创建商品列表、自定义字体和图标、滚轮控制下滑、隐藏滚动条等功能:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class ProductListGUI extends JFrame implements MouseWheelListener {
private JPanel mainPanel;
private JScrollPane scrollPane;
private JPanel productListPanel;
private JButton addButton;
public ProductListGUI() {
initUI();
}
private void initUI() {
setTitle("Product List");
setSize(500, 500);
setLocationRelativeTo(null);
setDefaultCloseOperation(EXIT_ON_CLOSE);
mainPanel = new JPanel();
mainPanel.setLayout(new BorderLayout());
productListPanel = new JPanel();
productListPanel.setLayout(new BoxLayout(productListPanel, BoxLayout.Y_AXIS));
productListPanel.setBackground(Color.WHITE);
scrollPane = new JScrollPane(productListPanel);
scrollPane.setVerticalScrollBarPolicy(JScrollPane.VERTICAL_SCROLLBAR_NEVER);
scrollPane.setHorizontalScrollBarPolicy(JScrollPane.HORIZONTAL_SCROLLBAR_NEVER);
scrollPane.getVerticalScrollBar().setUnitIncrement(16);
scrollPane.setBorder(BorderFactory.createEmptyBorder());
addButton = new JButton("Add Product");
addButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
addProduct();
}
});
mainPanel.add(scrollPane, BorderLayout.CENTER);
mainPanel.add(addButton, BorderLayout.SOUTH);
setContentPane(mainPanel);
addMouseWheelListener(this);
}
private void addProduct() {
JLabel productLabel = new JLabel("Product");
Font font = new Font("Arial", Font.BOLD, 18);
productLabel.setFont(font);
ImageIcon icon = new ImageIcon("product.png");
JLabel iconLabel = new JLabel(icon);
JPanel productPanel = new JPanel();
productPanel.setLayout(new FlowLayout(FlowLayout.LEFT));
productPanel.add(iconLabel);
productPanel.add(productLabel);
productPanel.setMaximumSize(new Dimension(Integer.MAX_VALUE, productPanel.getPreferredSize().height));
productPanel.setAlignmentX(Component.LEFT_ALIGNMENT);
productListPanel.add(productPanel);
productListPanel.revalidate();
productListPanel.repaint();
}
@Override
public void mouseWheelMoved(MouseWheelEvent e) {
int scrollAmount = scrollPane.getVerticalScrollBar().getUnitIncrement() * 3;
int scroll = scrollPane.getVerticalScrollBar().getValue() - e.getWheelRotation() * scrollAmount;
scrollPane.getVerticalScrollBar().setValue(scroll);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
ProductListGUI gui = new ProductListGUI();
gui.setVisible(true);
}
});
}
}
```
这个界面包含了一个主面板mainPanel,里面包含了一个商品列表面板productListPanel和一个添加商品按钮addButton。商品列表面板使用了BoxLayout,可以自适应高度,而添加商品按钮则放在了底部。商品列表面板使用了JScrollPane来实现滚动功能,水平和垂直滚动条都被隐藏。商品列表面板里的每个商品都是一个JPanel,包含了一个商品图标和一个商品名称,同时使用了自定义的字体。当用户点击添加商品按钮时,会在商品列表面板中添加一个新的商品。
注意,为了实现鼠标滚轮下滑功能,我们需要在ProductListGUI类中实现MouseWheelListener接口,并将自身添加为鼠标滚轮监听器。当用户滚动鼠标滚轮时,会触发mouseWheelMoved方法,我们可以在这个方法中计算滚动距离,并将滚动条的位置更新。
阅读全文
相关推荐
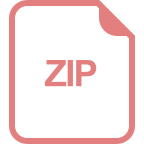

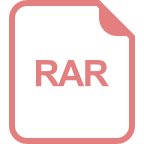
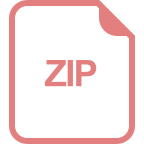
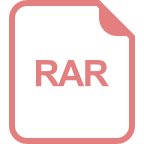
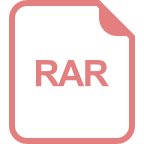
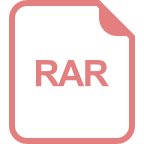
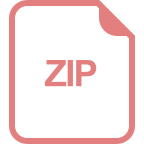
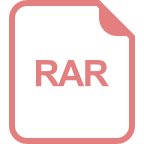
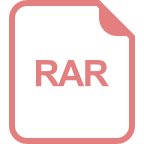
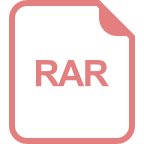
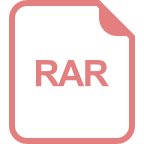
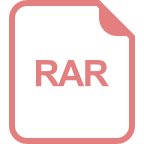
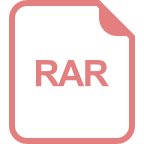