python KNN算法示例
时间: 2023-11-01 09:58:50 浏览: 49
kNN算法是一种常用的机器学习算法,用于分类和回归问题。下面是一个纯Python实现的kNN算法示例:
首先,导入需要的库和模块:
```python
import numpy as np
import operator
from os import listdir
```
然后,定义一个kNN分类函数:
```python
def classify(inputX, dataSet, labels, k):
# 计算输入样本与所有训练样本的距离
dataSize = dataSet.shape = classCount.get(voteLabel, 0) + 1
# 根据投票结果,返回分类结果
sortedClassCount = sorted(classCount.items(), key=operator.itemgetter(1), reverse=True)
return sortedClassCount
相关问题
python实现knn算法案例_Python实现的knn算法示例
以下是 Python 实现的 KNN 算法示例:
```python
import numpy as np
class KNN:
def __init__(self, k=3):
self.k = k
def fit(self, X, y):
self.X = X
self.y = y
def predict(self, X):
y_pred = []
for x in X:
distances = np.sqrt(np.sum((self.X - x)**2, axis=1))
indices = np.argsort(distances)[:self.k]
labels = self.y[indices]
y_pred.append(max(labels, key=list(labels).count))
return y_pred
```
其中,`KNN` 类有三个方法:
- `__init__(self, k=3)`:初始化方法,参数 `k` 为最近邻的个数,默认为 3。
- `fit(self, X, y)`:训练方法,参数 `X` 为训练数据,`y` 为标签。
- `predict(self, X)`:预测方法,参数 `X` 为测试数据,返回预测结果。
在该实现中,使用了 `numpy` 库计算欧氏距离和排序。`np.argsort()` 函数返回数组中元素从小到大排序后对应的索引,因此取前 `k` 个索引即为最近邻的索引。最后,返回最近邻中出现最多的标签作为预测结果。
Python实现knn算法
K最近邻(K-nearest neighbors,KNN)算法是一种常用的机器学习算法,用于分类和回归问题。下面是Python实现KNN算法的基本步骤:
1. 导入必要的库:首先,需要导入NumPy和Scikit-learn库。
```python
import numpy as np
from sklearn.neighbors import KNeighborsClassifier
```
2. 准备数据集:将数据集分为特征集和标签集。特征集包含用于分类的各个特征,而标签集包含对应的类别标签。
```python
# 示例数据集
X = np.array([[1, 2], [1, 4], [2, 2], [2, 3]])
y = np.array([0, 0, 1, 1])
```
3. 创建KNN分类器:使用Scikit-learn库中的KNeighborsClassifier类创建KNN分类器,并设置K值。
```python
# 创建KNN分类器,设置K值为3
knn = KNeighborsClassifier(n_neighbors=3)
```
4. 拟合数据:使用fit()方法将数据集拟合到KNN分类器中。
```python
# 拟合数据
knn.fit(X, y)
```
5. 进行预测:使用predict()方法对新样本进行分类预测。
```python
# 预测新样本
X_test = np.array([[3, 2], [1, 5]])
y_pred = knn.predict(X_test)
```
以上就是Python实现KNN算法的基本步骤。你可以根据自己的数据集和需求进行相应的调整和扩展。
相关推荐
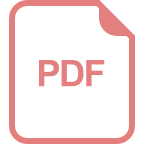
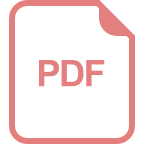
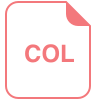
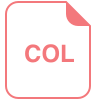
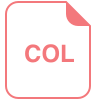
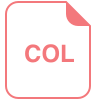
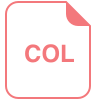







