实现Caesar密码(加法密码)加解密。明密文均可以是任意ASCII打印字符
时间: 2023-06-10 20:03:45 浏览: 151
好的,以下是实现Caesar密码(加法密码)加解密的Python代码示例:
加密:
```python
def caesar_encrypt(plaintext, shift):
ciphertext = ""
for c in plaintext:
if c.isprintable():
# 使用 ord() 方法把字符转换为 ASCII 码,加上偏移量 shift
# 使用 chr() 方法把加密后的 ASCII 码转换为字符
ciphertext += chr((ord(c) + shift) % 256)
else:
# 不是 ASCII 可打印字符,则直接添加该字符
ciphertext += c
return ciphertext
```
解密:
```python
def caesar_decrypt(ciphertext, shift):
plaintext = ""
for c in ciphertext:
if c.isprintable():
# 原理与加密相同,只是反向计算 ASCII 码
plaintext += chr((ord(c) - shift) % 256)
else:
plaintext += c
return plaintext
```
示例使用:
```python
plaintext = "Hello, world!"
shift = 3
ciphertext = caesar_encrypt(plaintext, shift)
# 加密后的密文为 "Khoor, zruog!"
print(ciphertext)
decoded_text = caesar_decrypt(ciphertext, shift)
# 解密后的明文为 "Hello, world!"
print(decoded_text)
```
上面的代码示例中,我们传入明文 plaintext 和偏移量 shift,得到了加密后的密文 ciphertext,再使用密文和相同的偏移量解密出原来的明文 decoded_text。
阅读全文
相关推荐

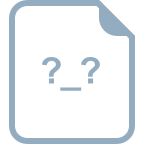
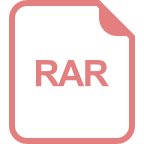
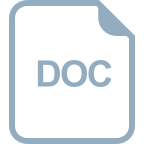

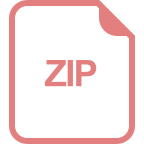
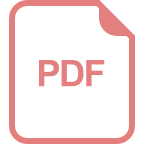
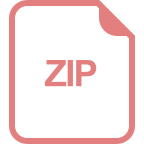
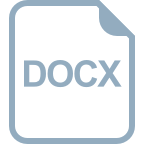
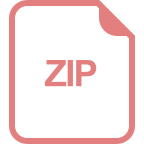
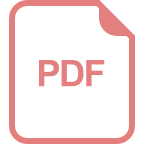
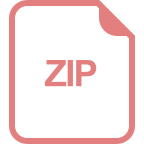