c++map怎么用匿名函数重载排序规则
时间: 2024-11-15 22:14:15 浏览: 5
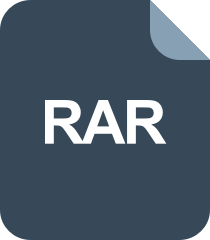
c c++函数手册.rar
在C++中,`std::map` 默认使用键的 `<` 运算符进行排序。如果你想使用自定义的排序规则,可以通过提供一个比较函数对象(或匿名函数)来实现。
以下是如何使用匿名函数重载 `std::map` 的排序规则的示例:
```cpp
#include <iostream>
#include <map>
#include <functional> // for std::greater
int main() {
// 使用 std::greater 作为比较函数对象,实现降序排序
std::map<int, std::string, std::greater<int>> myMap;
myMap[1] = "one";
myMap[2] = "two";
myMap[3] = "three";
// 输出 map 的内容
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
```
在这个例子中,我们使用了 `std::greater<int>` 作为比较函数对象,使得 `std::map` 按照键的降序排列。如果你想要更复杂的排序规则,可以定义自己的比较函数对象。例如:
```cpp
#include <iostream>
#include <map>
struct CustomCompare {
bool operator()(const int& lhs, const int& rhs) const {
// 自定义排序规则:偶数优先于奇数,且按降序排列
if ((lhs % 2 == 0) && (rhs % 2 != 0)) return true;
if ((lhs % 2 != 0) && (rhs % 2 == 0)) return false;
return lhs > rhs;
}
};
int main() {
std::map<int, std::string, CustomCompare> myMap;
myMap[1] = "one";
myMap[2] = "two";
myMap[3] = "three";
myMap[4] = "four";
// 输出 map 的内容
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
```
在这个例子中,我们定义了一个 `CustomCompare` 结构体,并重载了 `operator()` 以实现自定义的排序规则。然后我们将这个比较函数对象传递给 `std::map`,从而实现了自定义排序。
阅读全文
相关推荐
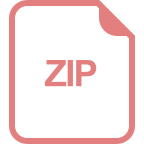
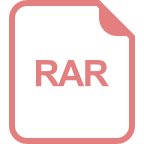

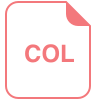
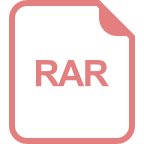
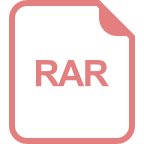
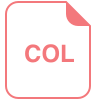
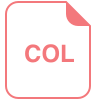

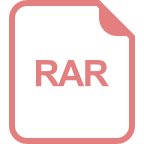
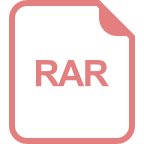
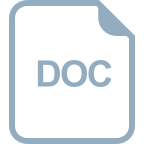
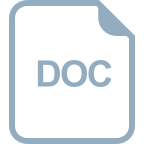
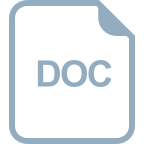
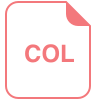
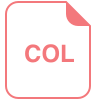
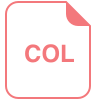
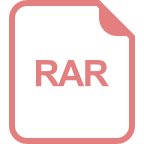