c# 多线程中 pictureBox图片上显示红色ROI
时间: 2023-06-27 20:02:29 浏览: 218
要在pictureBox中显示红色ROI,你需要在多线程中使用`Invoke`方法更新UI。
以下是一个示例代码:
```csharp
private void UpdateROI(Rectangle roi)
{
if (pictureBox1.InvokeRequired)
{
pictureBox1.Invoke((MethodInvoker)delegate ()
{
UpdateROI(roi);
});
}
else
{
using (Graphics g = pictureBox1.CreateGraphics())
{
Pen pen = new Pen(Color.Red, 2);
g.DrawRectangle(pen, roi);
}
}
}
```
在这个示例中,`UpdateROI`方法会在多线程中被调用,如果当前线程不是UI线程,那么将使用`Invoke`方法在UI线程上执行`UpdateROI`方法。
在UI线程上,使用`CreateGraphics`方法创建一个Graphics对象,并使用`DrawRectangle`方法绘制一个红色边框的矩形。
你可以在你的代码中调用`UpdateROI`方法来更新pictureBox中的ROI。
相关问题
c# 多线程中通button pictureBox图片上显示红色ROI
在 C# 多线程中,你需要使用 Invoke 方法将 UI 操作委托给 UI 线程。以下是一个示例代码,展示了如何在多线程中更新 PictureBox 的图片,并在图片上显示红色的矩形 ROI。
```csharp
private void StartButton_Click(object sender, EventArgs e)
{
// Start a new thread
Thread thread = new Thread(new ThreadStart(UpdatePictureBox));
thread.Start();
}
private void UpdatePictureBox()
{
// Load the image
Bitmap bmp = new Bitmap("image.jpg");
// Create a Graphics object from the PictureBox
Graphics g = pictureBox1.CreateGraphics();
// Draw the image on the PictureBox
pictureBox1.Invoke(new Action(() => { pictureBox1.Image = bmp; }));
// Draw a red rectangle on the PictureBox
Pen pen = new Pen(Color.Red, 2);
Rectangle rect = new Rectangle(100, 100, 50, 50);
g.Invoke(new Action(() => { g.DrawRectangle(pen, rect); }));
}
```
在这个示例中,当用户点击按钮时,会启动一个新的线程来更新 PictureBox 的图片。在 UpdatePictureBox 方法中,首先加载图片并将其绘制到 PictureBox 上。然后,使用 Graphics 对象在图片上绘制一个红色的矩形 ROI。
请注意,在更新 PictureBox 的图片和绘制矩形 ROI 时,都使用了 Invoke 方法将 UI 操作委托给 UI 线程。这是因为 UI 控件只能在 UI 线程中进行操作,如果在其他线程中访问它们,会引发异常。因此,使用 Invoke 方法可以确保这些操作在正确的线程中执行。
c# pictureBox图片上显示红色ROI
要在 C# 的 PictureBox 上显示红色的 ROI(感兴趣区域),可以使用 Graphics 对象的 DrawRectangle 方法来绘制一个矩形,并指定 Pen 对象的颜色为红色。以下是一个简单的示例代码:
```csharp
private void pictureBox1_Paint(object sender, PaintEventArgs e)
{
// 绘制一个红色的矩形
Pen pen = new Pen(Color.Red, 2);
Rectangle rect = new Rectangle(50, 50, 100, 100);
e.Graphics.DrawRectangle(pen, rect);
}
```
在此示例中,我们在 PictureBox 的 Paint 事件中绘制了一个红色的矩形,该事件会在 PictureBox 绘制时自动触发。您可以根据需要调整矩形的位置和大小。
阅读全文
相关推荐

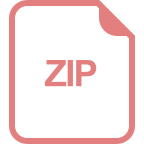






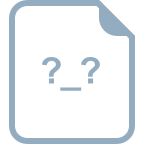




