展示分类结果的混淆矩阵;给出 F1-score 的定义,为什么很多时候使用 F1-score 的评价指标比准确率更好?对于该苹果分类器,准确率和 F1-score 哪个评价指 标更好?
时间: 2023-12-06 18:42:32 浏览: 137
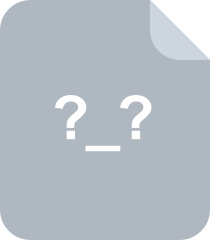
分类器的性能指标,混淆矩阵,Accuracy与recall等
混淆矩阵是一种用于可视化分类模型性能的表格,它将预测的类别与真实的类别进行比较,并将它们分为四个类别:真正例(True Positive, TP)、假正例(False Positive, FP)、真反例(True Negative, TN)和假反例(False Negative, FN)。其中,真正例是指模型正确预测为正例的样本数,假正例是指模型错误地将负例预测为正例的样本数,真反例是指模型正确预测为负例的样本数,假反例是指模型错误地将正例预测为负例的样本数。
F1-score是分类器的一个综合性度量,它同时考虑了分类器的精确率(precision)和召回率(recall),其定义为F1-score=2*precision*recall/(precision+recall)。精确率是指分类器预测为正例的样本中有多少是真正的正例,召回率是指所有真正的正例中有多少被分类器预测为正例。F1-score综合了这两个指标,因此可以更全面地评估分类器的性能。
在某些情况下,使用F1-score比准确率更好的原因是因为准确率不能很好地处理不平衡类别问题。当数据集中某个类别的样本数量很少时,分类器可能会倾向于将所有的样本都预测为多数类别,这会导致准确率很高,但是分类器的预测结果并不好。而F1-score在计算时同时考虑了精确率和召回率,因此可以更好地处理这种情况。
对于该苹果分类器,如果我们希望在多个类别之间进行评估,那么F1-score是更好的评价指标,因为它可以更全面地评估分类器的性能。而如果我们只关注分类器正确预测的样本比例,那么准确率可能更适合作为评价指标。
阅读全文
相关推荐
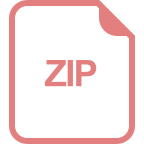
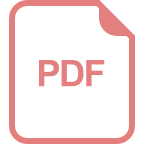
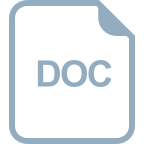
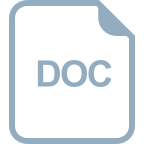
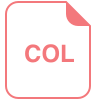
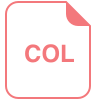











