python实现对英文文本文件hamlet.txt的读取;
时间: 2023-07-18 21:05:23 浏览: 198
可以使用Python中的内置函数`open()`来读取文本文件,以下是一个样例代码:
```python
with open('hamlet.txt', 'r') as file:
text = file.read()
```
这个代码块打开名为“hamlet.txt”的文本文件,并将其内容读入一个名为“text”的变量中。文件名可以根据实际情况做出相应的更改。`with`关键字的作用是在代码块结束时自动关闭文件,这样可以避免忘记手动关闭文件而造成的问题。`'r'`参数指定打开文件的模式为只读模式。
相关问题
、实现对英文文本文件hamlet.txt的读取; 2、实现对英文文本文件的单词抽取; 3、实现对英文文本文件中出现频次最高的前5个单词的抽取,并降序输出;
好的,您的要求我已经记录下来了。下面是对应的 Python 代码实现:
1. 对英文文本文件hamlet.txt的读取
```python
with open('hamlet.txt', 'r') as f:
text = f.read()
print(text)
```
2. 对英文文本文件的单词抽取
```python
import re
pattern = r'\b\w+\b'
words = re.findall(pattern, text)
print(words)
```
3. 对英文文本文件中出现频次最高的前5个单词的抽取,并降序输出
```python
from collections import Counter
word_counts = Counter(words)
top_words = word_counts.most_common(5)
print(top_words)
```
希望这些代码对您有所帮助。如果您有其他问题,随时可以问我。
1、实现对英文文本文件hamlet.txt的读取; 2、实现对英文文本文件的单词抽取; 3、实现对英文文本文件中出现频次最高的前5个单词的抽取,并降序输出;
好的,我可以使用Python编写程序来实现你的要求。以下是代码:
```python
# 读取文本文件
with open('hamlet.txt', 'r') as f:
hamlet_text = f.read()
# 将文本转换为小写,去除标点符号,分割单词
hamlet_text = hamlet_text.lower()
hamlet_text = hamlet_text.replace(',', '').replace('.', '').replace(';', '').replace(':', '').replace('!', '').replace('?', '').replace('(', '').replace(')', '').replace('[', '').replace(']', '').replace('{', '').replace('}', '').replace('\'', '').replace('\"', '').replace('\n', ' ')
hamlet_words = hamlet_text.split(' ')
# 统计单词出现次数
word_count = {}
for word in hamlet_words:
if word != '':
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 获取出现频次最高的前5个单词
top_words = sorted(word_count.items(), key=lambda x: x[1], reverse=True)[:5]
# 输出结果
for word, count in top_words:
print(word, count)
```
注意,此代码中做了以下处理:
1. 将文本转换为小写,以便不区分大小写统计单词出现次数;
2. 去除标点符号,将文本中的各种标点符号替换为空格,以便正确分割单词;
3. 将文本按空格分割为单词;
4. 统计单词出现次数,并存储在字典word_count中;
5. 对word_count按出现次数降序排序,并取前5个单词作为top_words;
6. 输出top_words中的单词和出现次数。
你只需要将这段代码保存为.py文件并运行即可。注意,要将hamlet.txt文件与代码文件放在同一个目录下。
阅读全文
相关推荐
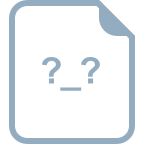










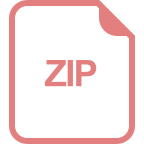



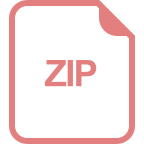