写一个用python压缩图片的代码
时间: 2023-12-17 16:45:50 浏览: 27
以下是一个用Python压缩图片的示例代码,使用了Pillow库:
```python
from PIL import Image
def compress_image(file_path, max_size):
"""压缩图片至指定大小以内"""
image = Image.open(file_path)
# 获取原图片尺寸
width, height = image.size
# 获取原图片大小(字节)
orig_size = image.size[0] * image.size[1] * 3
# 计算压缩比例
ratio = max_size / float(orig_size)
# 如果原图片已经小于指定大小,直接返回
if ratio >= 1:
return image
# 计算压缩后的尺寸
new_width = int(width * ratio)
new_height = int(height * ratio)
new_size = (new_width, new_height)
# 压缩图片
compressed_image = image.resize(new_size, Image.ANTIALIAS)
return compressed_image
# 测试代码
if __name__ == '__main__':
file_path = 'test.jpg'
max_size = 1024 * 1024 # 1MB
compressed_image = compress_image(file_path, max_size)
compressed_image.save('compressed.jpg')
```
在这个示例代码中,我们定义了一个 `compress_image()` 函数,接收要压缩的图片文件路径和压缩后的最大大小(单位:字节),并返回压缩后的图片对象。
该函数首先打开指定的图片,并获取其原尺寸和大小。然后,它计算出压缩比例,如果原图片已经小于指定大小,直接返回原图片;否则,计算出压缩后的尺寸,使用 `resize()` 方法压缩图片,最后返回压缩后的图片对象。
在测试代码中,我们指定了要压缩的图片文件路径和压缩后的最大大小,并将压缩后的图片保存到文件 `compressed.jpg` 中。
相关推荐
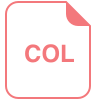

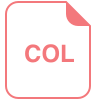
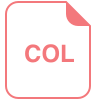













