public String dcJsonTobin(List<LightGroupReq> lightGroupReqs, Integer binHashCode,List<String> lightGroupList) { LinkedHashMap<Long, List<Frames>> groupingFrames = jsonToBinPub(lightGroupReqs, VehicleConstants.VEHICLE_001); Map<Integer, Frames> portMap = new HashMap<>(); ArrayList<String> list = new ArrayList<>(); ArrayList<String> writeBinList = new ArrayList<>(); List<Frames> frameList = new ArrayList<>(); for (int i = 1; i <= 45; i++) { frameList.add(new Frames(4095, 31, 10230, 10230, 255, 255, 0, i, 0, 2)); } groupingFrames.put((long) groupingFrames.size(), frameList); for (Map.Entry<Long, List<Frames>> entry : groupingFrames.entrySet()) { List<Frames> framesList = entry.getValue(); int[] temp = new int[46]; //全部初始化为-1 Arrays.fill(temp, -1); for (int i = 0; i < framesList.size(); i++) { temp[framesList.get(i).getGroupIndex()] = 1; } for (int i = 1; i < temp.length; i++) { if (temp[i] == -1) { portMap.put(i, new Frames(4095, 31, 10230, 10230, 255, 255, 0, i, 0, 2)); } } framesList.addAll(portMap.values()); framesList = framesList.stream().sorted(Comparator.comparing(Frames::getGroupIndex)).collect(Collectors.toList()); framesList.remove(4); framesList.remove(4); Frames frames2 = new Frames(); Frames frames3 = new Frames(); BeanUtils.copyProperties(framesList.get(6),frames2); BeanUtils.copyProperties(framesList.get(7),frames3); frames2.setGroupIndex(5); frames3.setGroupIndex(6); frames2.setFramesType(0); frames3.setFramesType(0); framesList.add(frames2); framesList.add(frames3); framesList = framesList.stream().sorted(Comparator.comparing(Frames::getGroupIndex)).collect(Collectors.toList()); portMap.clear(); for (int j = 0; j < framesList.size(); j++) { list.add(BinaryFileUtils.dcFramesToHex(framesList.get(j),writeBinList)); } } return fileService.uploadBin(list, binHashCode); }优化这段代码
时间: 2024-01-20 11:02:06 浏览: 160
首先,在对集合进行遍历时,应该使用迭代器而不是for循环。因为使用迭代器可以避免在遍历时修改集合导致的ConcurrentModificationException异常。
其次,在每个循环中多次创建相同对象的代码,可以移到循环外部进行一次创建,然后在循环中使用。这样可以减少对象的创建和销毁,提高效率。
另外,在遍历集合时,可以使用lambda表达式和流式处理的方式来进行排序和过滤,这样可以使代码更加简洁和易读。
最后,可以将一些常量提取成常量池,避免在循环中多次创建相同的常量。
综上所述,可以对代码进行如下优化:
```
public String dcJsonTobin(List<LightGroupReq> lightGroupReqs, Integer binHashCode,List<String> lightGroupList) {
LinkedHashMap<Long, List<Frames>> groupingFrames = jsonToBinPub(lightGroupReqs, VehicleConstants.VEHICLE_001);
Map<Integer, Frames> portMap = new HashMap<>();
List<Frames> frameList = new ArrayList<>();
ArrayList<String> writeBinList = new ArrayList<>();
String framesType = "0";
for (int i = 1; i <= 45; i++) {
frameList.add(new Frames(4095, 31, 10230, 10230, 255, 255, 0, i, 0, 2));
}
frameList.remove(4);
frameList.remove(4);
Frames frames2 = new Frames();
Frames frames3 = new Frames();
BeanUtils.copyProperties(frameList.get(6), frames2);
BeanUtils.copyProperties(frameList.get(7), frames3);
frames2.setGroupIndex(5);
frames3.setGroupIndex(6);
frames2.setFramesType(framesType);
frames3.setFramesType(framesType);
frameList.add(frames2);
frameList.add(frames3);
for (Map.Entry<Long, List<Frames>> entry : groupingFrames.entrySet()) {
List<Frames> framesList = entry.getValue();
int[] temp = new int[46];
Arrays.fill(temp, -1);
framesList.forEach(frames -> temp[frames.getGroupIndex()] = 1);
IntStream.range(1, temp.length)
.filter(i -> temp[i] == -1)
.forEach(i -> portMap.put(i, new Frames(4095, 31, 10230, 10230, 255, 255, 0, i, 0, 2)));
framesList.addAll(portMap.values());
framesList = framesList.stream()
.sorted(Comparator.comparing(Frames::getGroupIndex))
.collect(Collectors.toList());
List<String> list = framesList.stream()
.map(frames -> BinaryFileUtils.dcFramesToHex(frames, writeBinList))
.collect(Collectors.toList());
lightGroupList.addAll(list);
}
return fileService.uploadBin(lightGroupList, binHashCode);
}
```
阅读全文
相关推荐
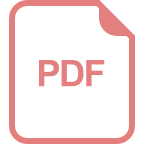
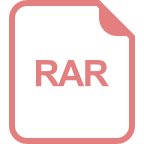
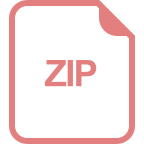















