import signal from time import sleep import serial #连接COM口 def connect(com,buad): ser = serial.Serial(com,buad,timeout=1) com_succes = "连接 {0} 成功".format(com) print(com_succes) ser.write(com_succes.encode('gbk')) ###################################################################### def if_input_data(): while True: # ser.write(b"ff") # sleep(1) count = ser.inWaiting() # 获取串口缓冲区数据 if count != 0: # sleep(0.1) #等待0.1秒 recv = ser.read(ser.in_waiting).decode("UTF-8") # 读出串口数据,数据采用gbk编码 print(recv) 如何把connect()函数中的ser 让第二个函数也能使用
时间: 2023-12-10 16:02:45 浏览: 33
可以将`ser`作为参数传递给`if_input_data()`函数,或者将`ser`定义为全局变量,这样就可以在不同的函数中使用它。
方法一:将`ser`作为参数传递给`if_input_data()`函数。
修改`if_input_data()`函数如下:
```python
def if_input_data(ser):
while True:
count = ser.inWaiting()
if count != 0:
recv = ser.read(ser.in_waiting).decode("UTF-8")
print(recv)
```
在`connect()`函数中调用`if_input_data()`函数时,将`ser`作为参数传递进去:
```python
def connect(com,buad):
ser = serial.Serial(com,buad,timeout=1)
com_succes = "连接 {0} 成功".format(com)
print(com_succes)
ser.write(com_succes.encode('gbk'))
if_input_data(ser) # 将ser作为参数传递给if_input_data函数
```
方法二:将`ser`定义为全局变量。
在`connect()`函数中将`ser`定义为全局变量:
```python
def connect(com,buad):
global ser # 定义ser为全局变量
ser = serial.Serial(com,buad,timeout=1)
com_succes = "连接 {0} 成功".format(com)
print(com_succes)
ser.write(com_succes.encode('gbk'))
```
然后在`if_input_data()`函数中使用`global`关键字声明`ser`为全局变量:
```python
def if_input_data():
global ser # 使用global关键字声明ser为全局变量
while True:
count = ser.inWaiting()
if count != 0:
recv = ser.read(ser.in_waiting).decode("UTF-8")
print(recv)
```
相关推荐
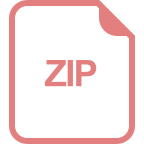





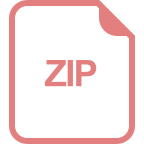
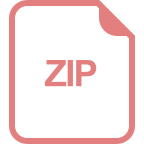
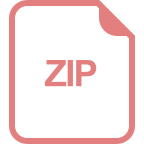
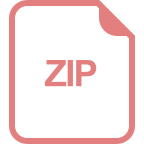
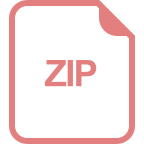
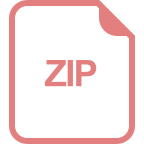
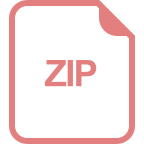