python多线程编程中,子线程绑定GPU失败,应该如何解决
时间: 2024-04-12 14:29:54 浏览: 223
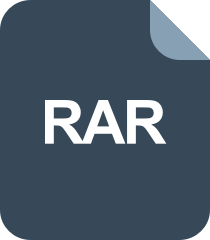
python 多线程编程
在 Python 多线程编程中,子线程绑定 GPU 失败的问题可以通过以下几种方式解决:
1. 在每个子线程中都调用 `torch.cuda.set_device()` 显式地设置要使用的 GPU 设备。这可以确保在每个子线程中使用的 CUDA 上下文与主线程中的一致。例如:
```python
import torch
import threading
def worker():
torch.cuda.set_device(0) # 设置要使用的 GPU 设备
# 在子线程中进行 GPU 相关的操作
# 创建子线程并启动
thread = threading.Thread(target=worker)
thread.start()
```
2. 将需要在子线程中进行的 GPU 相关操作放在一个函数中,然后通过 `torch.cuda.stream()` 创建一个新的 CUDA 流,并将操作放入该流中执行。这样可以避免多个线程之间的 CUDA 上下文冲突。例如:
```python
import torch
import threading
def worker():
# 创建一个新的 CUDA 流
stream = torch.cuda.stream()
with torch.cuda.stream(stream):
# 在子线程中进行 GPU 相关的操作
# 创建子线程并启动
thread = threading.Thread(target=worker)
thread.start()
```
3. 使用 `torch.cuda.device()` 上下文管理器手动管理 CUDA 上下文。这样可以确保在子线程结束时正确释放 CUDA 资源。例如:
```python
import torch
import threading
def worker():
with torch.cuda.device(0): # 设置要使用的 GPU 设备
# 在子线程中进行 GPU 相关的操作
# 创建子线程并启动
thread = threading.Thread(target=worker)
thread.start()
```
需要注意的是,多线程环境下使用 GPU 时,要确保正确地管理 CUDA 上下文,避免出现资源泄漏或冲突的问题。并且要根据具体情况选择适合的解决方案。
阅读全文
相关推荐
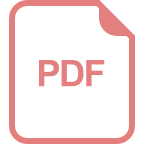




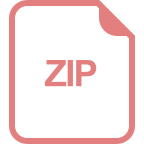



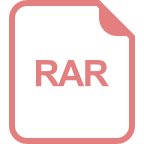
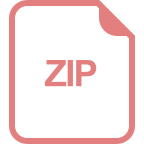
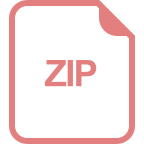