随机森林的具体案例关于python的
时间: 2023-11-26 10:33:44 浏览: 86
好的,下面给你一个用Python实现随机森林的案例。
案例:根据房屋的各种特征预测房屋的销售价格。
1. 数据预处理
首先,我们需要导入必要的库并读取数据集。在这个案例中,我们使用了Kaggle上的房屋销售价格数据集(https://www.kaggle.com/c/house-prices-advanced-regression-techniques)。
```python
import pandas as pd
from sklearn.model_selection import train_test_split
# 读取数据集
data = pd.read_csv('train.csv')
# 处理缺失值
data = data.fillna(data.mean())
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(data.drop('SalePrice', axis=1),
data['SalePrice'],
test_size=0.2,
random_state=42)
```
2. 特征工程
接下来,我们需要进行特征工程,即对原始数据进行处理以提取更有用的特征。在这个案例中,我们使用了One-Hot Encoding来对类别特征进行处理。
```python
from sklearn.preprocessing import OneHotEncoder
# 处理类别特征
cat_features = ['MSSubClass', 'MSZoning', 'Street', 'Alley', 'LotShape',
'LandContour', 'Utilities', 'LotConfig', 'LandSlope',
'Neighborhood', 'Condition1', 'Condition2', 'BldgType',
'HouseStyle', 'RoofStyle', 'RoofMatl', 'Exterior1st',
'Exterior2nd', 'MasVnrType', 'ExterQual', 'ExterCond',
'Foundation', 'BsmtQual', 'BsmtCond', 'BsmtExposure',
'BsmtFinType1', 'BsmtFinType2', 'Heating', 'HeatingQC',
'CentralAir', 'Electrical', 'KitchenQual', 'Functional',
'FireplaceQu', 'GarageType', 'GarageFinish', 'GarageQual',
'GarageCond', 'PavedDrive', 'PoolQC', 'Fence', 'MiscFeature',
'SaleType', 'SaleCondition']
encoder = OneHotEncoder(handle_unknown='ignore')
encoder.fit(X_train[cat_features])
# 转换训练集和测试集
X_train_encoded = encoder.transform(X_train[cat_features])
X_test_encoded = encoder.transform(X_test[cat_features])
# 将编码后的类别特征和数值特征合并
X_train_final = pd.concat([pd.DataFrame(X_train_encoded.toarray()),
X_train.drop(cat_features, axis=1)], axis=1)
X_test_final = pd.concat([pd.DataFrame(X_test_encoded.toarray()),
X_test.drop(cat_features, axis=1)], axis=1)
```
3. 训练模型
现在,我们已经准备好训练随机森林模型了。在这个案例中,我们使用了sklearn库中的RandomForestRegressor。
```python
from sklearn.ensemble import RandomForestRegressor
from sklearn.metrics import mean_squared_error
# 训练随机森林模型
rf = RandomForestRegressor(n_estimators=100, max_depth=10, random_state=42)
rf.fit(X_train_final, y_train)
# 在测试集上进行预测
y_pred = rf.predict(X_test_final)
# 计算均方根误差
rmse = mean_squared_error(y_test, y_pred, squared=False)
print('RMSE:', rmse)
```
4. 模型优化
最后,我们可以进行模型优化。在这个案例中,我们使用了Grid Search来寻找最优的超参数。
```python
from sklearn.model_selection import GridSearchCV
# 定义参数范围
params = {'n_estimators': [50, 100, 200], 'max_depth': [5, 10, 20]}
# 使用Grid Search进行参数搜索
grid_search = GridSearchCV(RandomForestRegressor(random_state=42), params, cv=5)
grid_search.fit(X_train_final, y_train)
# 输出最优参数
print('Best params:', grid_search.best_params_)
# 在测试集上进行预测
y_pred_optimized = grid_search.predict(X_test_final)
# 计算均方根误差
rmse_optimized = mean_squared_error(y_test, y_pred_optimized, squared=False)
print('Optimized RMSE:', rmse_optimized)
```
以上就是一个简单的随机森林案例。在实际应用中,需要根据具体问题进行数据预处理、特征工程和模型优化等步骤。
阅读全文
相关推荐
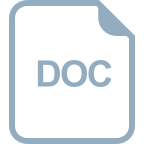
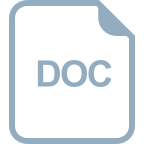
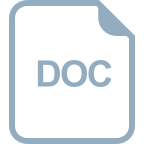
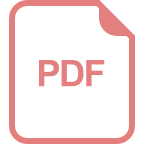
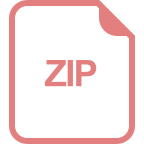
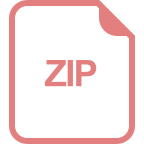
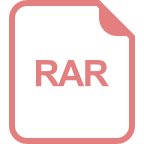
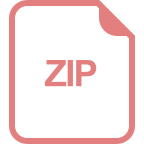
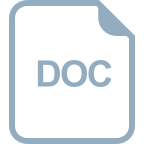







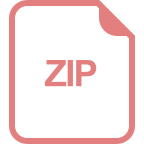
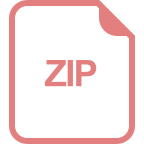