写一个C语言实现的物体分类器
时间: 2024-02-03 18:14:40 浏览: 25
物体分类器是一种基于机器学习的应用程序,它可以将输入的图像归类为不同的物体类型。在这里,我们使用C语言实现一个简单的物体分类器,基于一个预先训练好的模型,并使用OpenCV库来处理图像。
首先,我们需要安装OpenCV库。可以使用以下命令在Ubuntu上进行安装:
```
sudo apt-get update
sudo apt-get install libopencv-dev
```
接下来,我们需要加载预先训练好的模型。这里我们使用一个已经训练好的模型,名为"res10_300x300_ssd_iter_140000_fp16.caffemodel",可以从Github上下载。
```c
#include <opencv2/opencv.hpp>
#include <opencv2/dnn.hpp>
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
using namespace cv;
using namespace cv::dnn;
using namespace std;
// 加载预训练的模型
Net load_model() {
String modelTxt = "deploy.prototxt";
String modelBin = "res10_300x300_ssd_iter_140000_fp16.caffemodel";
Net net = readNetFromCaffe(modelTxt, modelBin);
return net;
}
```
接下来,我们需要定义一个函数,该函数将读取图像并使用模型进行分类。
```c
// 对图像进行分类
void classify_image(const Mat& img, Net net) {
Mat inputBlob = blobFromImage(img, 1.0, Size(300, 300), Scalar(104.0, 177.0, 123.0), false, false);
net.setInput(inputBlob, "data");
Mat detection = net.forward("detection_out");
Mat detectionMat(detection.size[2], detection.size[3], CV_32F, detection.ptr<float>());
for (int i = 0; i < detectionMat.rows; i++) {
float confidence = detectionMat.at<float>(i, 2);
int classId = detectionMat.at<float>(i, 1);
// 如果置信度超过0.5,就认为是一个有效的检测
if (confidence > 0.5) {
// 获取检测框的坐标
int xLeftBottom = static_cast<int>(detectionMat.at<float>(i, 3) * img.cols);
int yLeftBottom = static_cast<int>(detectionMat.at<float>(i, 4) * img.rows);
int xRightTop = static_cast<int>(detectionMat.at<float>(i, 5) * img.cols);
int yRightTop = static_cast<int>(detectionMat.at<float>(i, 6) * img.rows);
// 画出检测框
Rect rect(xLeftBottom, yLeftBottom, xRightTop - xLeftBottom, yRightTop - yLeftBottom);
rectangle(img, rect, Scalar(0, 255, 0), 2);
// 获取物体类别标签
stringstream ss;
ss << confidence;
String conf(ss.str());
String label = format("Class %d: %s", classId, conf.c_str());
int baseline;
Size labelSize = getTextSize(label, FONT_HERSHEY_SIMPLEX, 0.5, 1, &baseline);
rectangle(img, Point(xLeftBottom, yLeftBottom - labelSize.height),
Point(xLeftBottom + labelSize.width, yLeftBottom + baseline), Scalar(255, 255, 255), FILLED);
putText(img, label, Point(xLeftBottom, yLeftBottom), FONT_HERSHEY_SIMPLEX, 0.5, Scalar(0, 0, 0));
}
}
imshow("Classification", img);
waitKey(0);
}
```
最后,我们编写主函数,该函数将读取图像并调用分类函数进行分类。
```c
int main(int argc, char** argv) {
// 加载预训练的模型
Net net = load_model();
// 读取图像并进行分类
Mat img = imread("image.jpg");
classify_image(img, net);
return 0;
}
```
上述代码将读取名为"image.jpg"的图像,并使用预先训练的模型对其进行分类。分类结果将显示在窗口中。
请注意,这只是一个简单的物体分类器示例,可以通过改进模型或使用更复杂的算法来提高分类器的准确性。
相关推荐
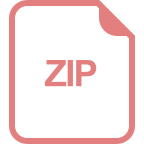















