使用C++实现AES算法进程实现
时间: 2024-04-08 17:07:24 浏览: 106
以下是使用C++语言实现AES算法的过程:
1. 定义AES算法的常量
```cpp
const int Nb = 4; // 每个状态矩阵的列数
const int Nk = 4; // 密钥矩阵的列数
const int Nr = 10; // 加密轮数
```
2. 定义AES算法的数据类型
```cpp
typedef unsigned char byte; // 一个字节(8位)
typedef unsigned int word; // 一个字(32位)
```
3. 定义AES算法的核心函数
```cpp
void SubBytes(byte state[][Nb]); // 字节替换
void ShiftRows(byte state[][Nb]); // 行移位
void MixColumns(byte state[][Nb]); // 列混淆
void AddRoundKey(byte state[][Nb], word w[]); // 轮密钥加
void KeyExpansion(byte key[], word w[]); // 密钥扩展
void Cipher(byte input[], byte output[], word w[]); // 加密
```
4. 实现AES算法的主函数
```cpp
int main()
{
byte key[] = { ... }; // 密钥
byte input[] = { ... }; // 明文
byte output[16]; // 密文
word w[Nb * (Nr + 1)]; // 扩展密钥
KeyExpansion(key, w);
Cipher(input, output, w);
return 0;
}
```
5. 实现AES算法的核心函数
```cpp
// S盒
const byte Sbox[256] = { ... };
// 逆S盒
const byte InvSbox[256] = { ... };
// Rcon
const byte Rcon[11] = { 0x00, 0x01, 0x02, 0x04, 0x08, 0x10, 0x20, 0x40, 0x80, 0x1b, 0x36 };
// 字节替换
void SubBytes(byte state[][Nb])
{
for (int i = 0; i < 4; i++)
{
for (int j = 0; j < Nb; j++)
{
state[i][j] = Sbox[state[i][j]];
}
}
}
// 行移位
void ShiftRows(byte state[][Nb])
{
byte tmp;
// 第二行循环左移1位
tmp = state[1][0];
state[1][0] = state[1][1];
state[1][1] = state[1][2];
state[1][2] = state[1][3];
state[1][3] = tmp;
// 第三行循环左移2位
tmp = state[2][0];
state[2][0] = state[2][2];
state[2][2] = tmp;
tmp = state[2][1];
state[2][1] = state[2][3];
state[2][3] = tmp;
// 第四行循环左移3位
tmp = state[3][0];
state[3][0] = state[3][3];
state[3][3] = state[3][2];
state[3][2] = state[3][1];
state[3][1] = tmp;
}
// 列混淆
void MixColumns(byte state[][Nb])
{
byte tmp[Nb];
for (int i = 0; i < Nb; i++)
{
tmp[0] = (byte)(GF28Mul(0x02, state[0][i]) ^ GF28Mul(0x03, state[1][i]) ^ state[2][i] ^ state[3][i]);
tmp[1] = (byte)(state[0][i] ^ GF28Mul(0x02, state[1][i]) ^ GF28Mul(0x03, state[2][i]) ^ state[3][i]);
tmp[2] = (byte)(state[0][i] ^ state[1][i] ^ GF28Mul(0x02, state[2][i]) ^ GF28Mul(0x03, state[3][i]));
tmp[3] = (byte)(GF28Mul(0x03, state[0][i]) ^ state[1][i] ^ state[2][i] ^ GF28Mul(0x02, state[3][i]));
for (int j = 0; j < Nb; j++)
{
state[j][i] = tmp[j];
}
}
}
// 轮密钥加
void AddRoundKey(byte state[][Nb], word w[])
{
for (int i = 0; i < Nb; i++)
{
word tmp = (w[i] >> 24) << 24 | (w[i] >> 16) << 16 | (w[i] >> 8) << 8 | w[i];
byte* p = (byte*)&tmp;
state[0][i] ^= p[0];
state[1][i] ^= p[1];
state[2][i] ^= p[2];
state[3][i] ^= p[3];
}
}
// 密钥扩展
void KeyExpansion(byte key[], word w[])
{
word tmp;
// 第一轮
for (int i = 0; i < Nk; i++)
{
w[i] = (key[4 * i] << 24) | (key[4 * i + 1] << 16) | (key[4 * i + 2] << 8) | key[4 * i + 3];
}
// 后面的轮
for (int i = Nk; i < Nb * (Nr + 1); i++)
{
tmp = w[i - 1];
if (i % Nk == 0)
{
tmp = (Sbox[(tmp >> 16) & 0xff] << 24) | (Sbox[(tmp >> 8) & 0xff] << 16) | (Sbox[tmp & 0xff] << 8) | Sbox[(tmp >> 24) & 0xff];
tmp ^= Rcon[i / Nk] << 24;
}
else if (Nk > 6 && i % Nk == 4)
{
tmp = (Sbox[(tmp >> 24) & 0xff] << 24) | (Sbox[(tmp >> 16) & 0xff] << 16) | (Sbox[(tmp >> 8) & 0xff] << 8) | Sbox[tmp & 0xff];
}
w[i] = w[i - Nk] ^ tmp;
}
}
// 加密
void Cipher(byte input[], byte output[], word w[])
{
byte state[Nb][Nb];
// 将明文分组成状态矩阵
for (int i = 0; i < Nb; i++)
{
for (int j = 0; j < Nb; j++)
{
state[j][i] = input[i * Nb + j];
}
}
// 轮密钥加
AddRoundKey(state, w);
// Nr-1轮
for (int round = 1; round < Nr; round++)
{
SubBytes(state);
ShiftRows(state);
MixColumns(state);
AddRoundKey(state, w + round * Nb);
}
// 最后一轮
SubBytes(state);
ShiftRows(state);
AddRoundKey(state, w + Nr * Nb);
// 将密文从状态矩阵中提取出来
for (int i = 0; i < Nb; i++)
{
for (int j = 0; j < Nb; j++)
{
output[i * Nb + j] = state[j][i];
}
}
}
```
以上就是使用C++实现AES算法的过程。需要注意的是,这里的实现仅供参考,可能存在一些问题,例如未对输入进行检查,可能导致溢出等问题。在实际应用中,需要根据具体情况进行改进和调整。
阅读全文
相关推荐
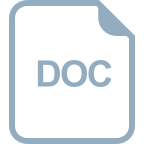
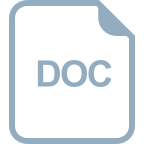
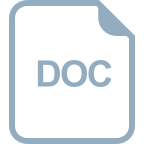
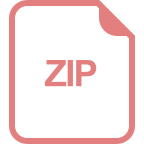
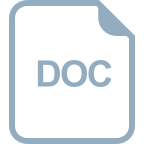
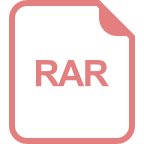
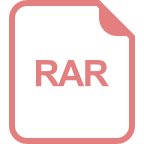
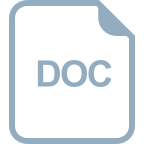
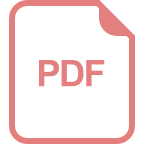
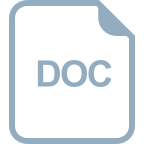
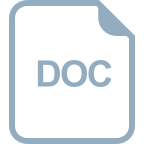
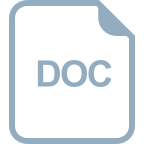
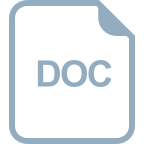
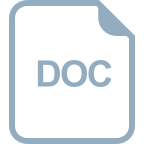
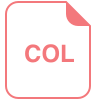
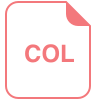
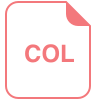

