Consider two 4-bit binary numbers A and B. Bits of A are a3, a2, a1, and a0, and bits of B are b3, b2, b1, and b0. A is greater than B if a3 is 1 and b3 is 0, but if a3 and b3 are the same, then if a2 is 1 and b2 is 0, we can determine that A is greater than B. This evaluation continues until a0 and b0 are considered. If a0 and b0 are equal then A and B are equal. Using discrete gates and Verilog gate primitives build a 4-bit comparator that generates a 1 on its GT output when its 4-bit input A is greater than its B input, and a 1 on its EQ output when A and B are equal. Use the generate statement and other Verilog iterative logic support structures.
时间: 2023-12-24 19:02:42 浏览: 17
Here is a Verilog implementation of the 4-bit comparator:
```
module comparator (
input [3:0] A,
input [3:0] B,
output reg GT,
output reg EQ
);
// check if A is greater than B
always @(*) begin
GT = 0;
EQ = 0;
if (A[3] == 1 && B[3] == 0) begin
GT = 1;
end else if (A[3] == B[3]) begin
if (A[2] == 1 && B[2] == 0) begin
GT = 1;
end else if (A[2] == B[2]) begin
if (A[1] == 1 && B[1] == 0) begin
GT = 1;
end else if (A[1] == B[1]) begin
if (A[0] == 1 && B[0] == 0) begin
GT = 1;
end else if (A[0] == B[0]) begin
EQ = 1;
end
end
end
end
end
endmodule
```
The module takes in two 4-bit inputs `A` and `B`, and outputs `GT` and `EQ`, which are 1 if `A` is greater than or equal to `B`, respectively.
The comparator uses a series of if-else statements to compare each bit of `A` and `B`, starting with the most significant bit. If `A` is greater than `B` at any point, the `GT` output is set to 1. If `A` is not greater than `B` at any point, but they are equal, then the `EQ` output is set to 1.
阅读全文
相关推荐
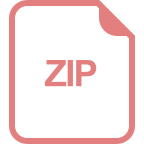
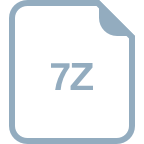
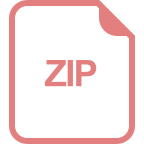
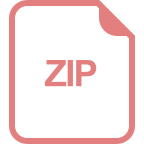
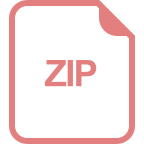
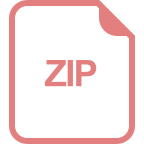
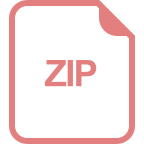