springboot登录密码加密
时间: 2023-11-16 11:52:55 浏览: 133
在Spring Boot中,可以使用一些加密算法来对登录密码进行加密。以下是一种常用的方法:
1. 导入相关依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
2. 创建一个密码编码器的 Bean:
```java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
@Configuration
public class SecurityConfig {
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
3. 在登录认证的逻辑中使用密码编码器:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.stereotype.Service;
@Service
public class AuthService {
@Autowired
private PasswordEncoder passwordEncoder;
public boolean authenticate(String username, String password) {
// 从数据库或其他存储中获取已保存的加密密码
String savedPassword = // 从数据库中获取已保存的加密密码
// 使用密码编码器验证密码
return passwordEncoder.matches(password, savedPassword);
}
}
```
4. 在注册新用户时,对密码进行加密处理:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Autowired
private PasswordEncoder passwordEncoder;
public void registerUser(String username, String password) {
// 对密码进行加密
String encryptedPassword = passwordEncoder.encode(password);
// 将加密后的密码保存到数据库或其他存储中
// ...
}
}
```
阅读全文
相关推荐
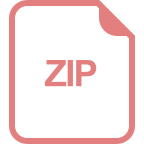

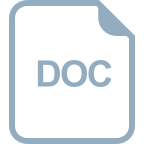


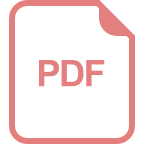
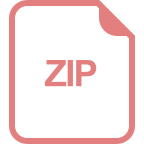
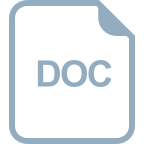








