如何使用 Picker 实现一个下拉框,有三个选型,每个选项还有三个子选项
时间: 2024-01-27 15:06:20 浏览: 23
要实现一个下拉框,可以使用 React Native 中的 Picker 组件。下面是一个具有三个选项,每个选项还有三个子选项的示例代码:
```javascript
import React, { useState } from 'react';
import { View, Picker } from 'react-native';
const options = [
{ label: 'Option 1', value: 'option1', children: [
{ label: 'Child 1', value: 'child1' },
{ label: 'Child 2', value: 'child2' },
{ label: 'Child 3', value: 'child3' },
]},
{ label: 'Option 2', value: 'option2', children: [
{ label: 'Child 4', value: 'child4' },
{ label: 'Child 5', value: 'child5' },
{ label: 'Child 6', value: 'child6' },
]},
{ label: 'Option 3', value: 'option3', children: [
{ label: 'Child 7', value: 'child7' },
{ label: 'Child 8', value: 'child8' },
{ label: 'Child 9', value: 'child9' },
]},
];
const MyPicker = () => {
const [selectedOption, setSelectedOption] = useState(options[0].value);
const [selectedChild, setSelectedChild] = useState(options[0].children[0].value);
const handleOptionChange = (value) => {
setSelectedOption(value);
setSelectedChild(options.find(option => option.value === value).children[0].value);
};
const handleChildChange = (value) => {
setSelectedChild(value);
};
const optionItems = options.map(option => (
<Picker.Item
key={option.value}
label={option.label}
value={option.value}
/>
));
const childItems = options.find(option => option.value === selectedOption).children.map(child => (
<Picker.Item
key={child.value}
label={child.label}
value={child.value}
/>
));
return (
<View>
<Picker selectedValue={selectedOption} onValueChange={handleOptionChange}>
{optionItems}
</Picker>
<Picker selectedValue={selectedChild} onValueChange={handleChildChange}>
{childItems}
</Picker>
</View>
);
};
export default MyPicker;
```
这个组件中有两个 Picker,一个用于选择选项,另一个用于选择子选项。选项和子选项都是通过 options 数组定义的。选项和子选项都是通过 Picker.Item 渲染的。在这个示例中,当选项改变时,子选项会自动切换到第一个子选项。
相关推荐
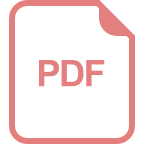
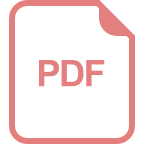
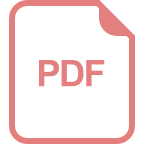














