QT实现在聊天室邀请其他用户的c++代码
时间: 2023-10-30 13:54:42 浏览: 43
以下是一个简单的 QT 聊天室客户端的代码,包含邀请其他用户的功能:
```cpp
#include <QTcpSocket>
#include <QLineEdit>
#include <QPushButton>
#include <QVBoxLayout>
#include <QHBoxLayout>
#include <QListWidget>
#include <QInputDialog>
#include <QMessageBox>
int main(int argc, char *argv[])
{
QTcpSocket socket;
QListWidget chatWidget;
// 连接到服务端
socket.connectToHost(QHostAddress::LocalHost, 12345);
// 界面布局
QLineEdit messageEdit;
QPushButton sendButton("发送");
QPushButton inviteButton("邀请");
QHBoxLayout bottomLayout;
bottomLayout.addWidget(&messageEdit);
bottomLayout.addWidget(&sendButton);
bottomLayout.addWidget(&inviteButton);
QVBoxLayout mainLayout;
mainLayout.addWidget(&chatWidget);
mainLayout.addLayout(&bottomLayout);
QWidget window;
window.setLayout(&mainLayout);
window.show();
// 发送消息
connect(&sendButton, &QPushButton::clicked, [=]() {
QString message = messageEdit.text();
if (!message.isEmpty()) {
socket.write(message.toUtf8());
messageEdit.clear();
}
});
// 邀请其他用户
connect(&inviteButton, &QPushButton::clicked, [=]() {
bool ok;
QString username = QInputDialog::getText(&window, "邀请用户", "请输入用户名:", QLineEdit::Normal, "", &ok);
if (ok && !username.isEmpty()) {
// 发送邀请消息
socket.write(QString("invite:%1").arg(username).toUtf8());
}
});
// 接收服务端发送的消息
connect(&socket, &QTcpSocket::readyRead, [=]() {
QByteArray message = socket.readAll();
// 如果是邀请消息,则弹出提示框
if (message.startsWith("invitation from ")) {
QString username = message.mid(16);
QMessageBox::information(&window, "收到邀请", QString("%1 邀请您加入聊天室!").arg(username));
}
// 否则将消息添加到聊天窗口
else {
chatWidget.addItem(message);
}
});
return 0;
}
```
在客户端发送邀请消息时,需要在消息前添加 "invite:" 前缀,服务端会解析该消息并向其他客户端广播邀请消息。
注意,该示例代码仅仅是一个简单的聊天室,如果需要更完善的聊天室功能,需要在服务端添加用户管理和权限控制等功能。
相关推荐
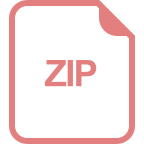
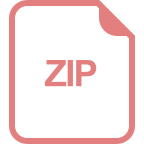
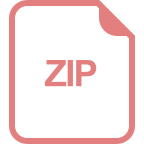
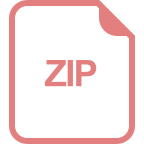
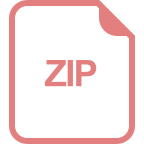
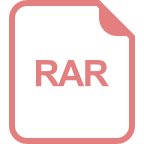
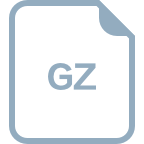
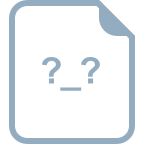
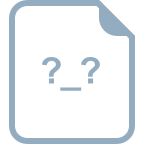
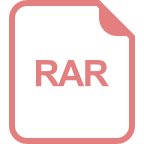
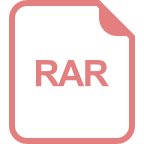
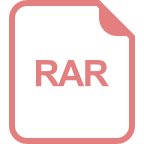
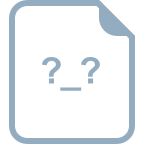
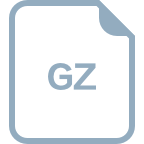