如何用springboot和RabbitMQ实现发送通知给用户的功能,可以给出示例代码吗
时间: 2024-02-24 12:53:43 浏览: 172
当用户发生某些操作时,我们可以使用RabbitMQ来异步发送通知给用户,以提高系统的性能和并发性。下面是一个使用Spring Boot和RabbitMQ实现发送通知给用户的示例代码:
首先,需要在Spring Boot中配置RabbitMQ,可以在application.properties文件中添加以下配置:
```
spring.rabbitmq.host=localhost
spring.rabbitmq.port=5672
spring.rabbitmq.username=guest
spring.rabbitmq.password=guest
```
然后,创建一个发送通知的服务类,例如NotificationService:
```java
@Service
public class NotificationService {
@Autowired
private RabbitTemplate rabbitTemplate;
public void sendNotification(String userId, String message) {
rabbitTemplate.convertAndSend("notification.exchange", "notification." + userId, message);
}
}
```
在上面的代码中,我们使用RabbitTemplate来发送消息,将通知发送到名为notification.exchange的交换机中,使用用户ID作为路由键,以便将通知发送到正确的用户队列中。
接下来,在应用程序启动时,我们需要创建名为notification.exchange的交换机和每个用户的队列。可以使用以下代码完成此操作:
```java
@Configuration
public class RabbitMQConfig {
@Bean
public TopicExchange notificationExchange() {
return new TopicExchange("notification.exchange");
}
@Bean
public Queue userQueue() {
return new Queue("notification.user.#");
}
@Bean
public Binding userBinding(TopicExchange notificationExchange, Queue userQueue) {
return BindingBuilder.bind(userQueue).to(notificationExchange).with("notification.user.#");
}
}
```
在上面的代码中,我们创建了一个名为notification.exchange的TopicExchange,以及一个名为notification.user.#的队列和一个绑定,用于将队列绑定到交换机上。
最后,我们可以在控制器中调用NotificationService来发送通知,例如:
```java
@RestController
public class UserController {
@Autowired
private NotificationService notificationService;
@PostMapping("/users/{userId}/do-something")
public ResponseEntity<?> doSomething(@PathVariable String userId) {
// do something
notificationService.sendNotification(userId, "Something has been done!");
return ResponseEntity.ok().build();
}
}
```
在上面的代码中,我们在用户执行某些操作后调用NotificationService来发送通知。
当用户需要接收通知时,可以使用RabbitMQ的客户端来监听其队列并处理通知。例如:
```java
@Component
public class UserNotificationReceiver {
@RabbitListener(queues = "notification.user.#{userId}")
public void receiveNotification(String message) {
// process notification
System.out.println("Received notification: " + message);
}
}
```
在上面的代码中,我们使用@RabbitListener注解来监听队列,以接收来自RabbitMQ的通知。每当有新的通知到达用户的队列时,该方法就会被调用,并且我们可以在其中处理通知。
这是一个简单的示例,说明如何使用Spring Boot和RabbitMQ发送通知给用户。当然,实际应用程序中可能需要更复杂的逻辑和处理。
阅读全文
相关推荐
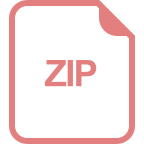
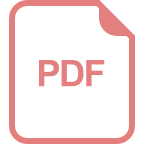
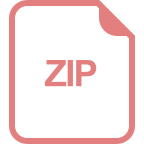









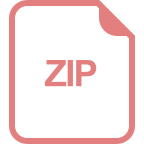
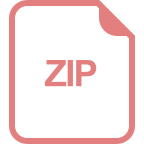
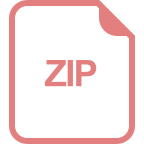
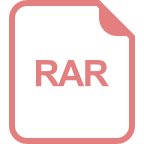
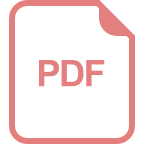
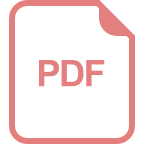
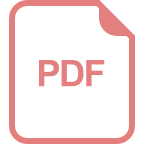
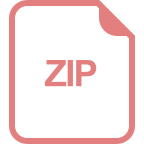