用遗传算法求解巡航高度优化问题,绘制适应度值、适应度平均值随迭代次数的变化情况。共有三个高度选项,分别为9200、9800、10400米;共有八个航段;起点高度设置为9800米;高度10400米的八个航段的油耗为[7, 7, 6, 11, 12, 5, 6, 9]、高度9800米的八个航段的油耗为[8, 9, 9, 7, 6, 9, 8, 3]、高度9200米的八个航段的油耗为[10, 10, 10, 10, 10, 10, 10, 10]
时间: 2023-09-09 15:06:31 浏览: 165
好的,我理解了你的问题。这是一个典型的优化问题,可以使用遗传算法求解。以下是我对这个问题的解决方案:
1. 遗传算法的编码方式
我们可以将一个飞行高度优化方案编码为一个长度为8的二进制串,每个二进制位表示对应航段的飞行高度选项。例如,001表示第一段飞行高度为9200米,第二段飞行高度为9800米,第三段飞行高度为10400米。
2. 遗传算法的参数设置
- 种群大小:50
- 交叉概率:0.6
- 变异概率:0.01
- 最大迭代次数:100
- 选择算子:锦标赛选择
3. 适应度函数的定义
我们可以将适应度函数定义为飞行方案的总油耗,即适应度值为每个航段油耗乘以对应航段的飞行高度选项的值之和。
4. 绘制适应度值、适应度平均值随迭代次数的变化情况
在每次迭代中,记录种群中适应度值最好的个体的适应度值和适应度平均值,并将其绘制成图表。下面是一个示例代码,使用Python语言实现了该算法的求解过程。
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义问题参数
HEIGHT_OPTIONS = [9200, 9800, 10400]
SEGMENTS = 8
START_HEIGHT = HEIGHT_OPTIONS[1]
OIL_CONSUMPTIONS = {
HEIGHT_OPTIONS[0]: [10, 10, 10, 10, 10, 10, 10, 10],
HEIGHT_OPTIONS[1]: [8, 9, 9, 7, 6, 9, 8, 3],
HEIGHT_OPTIONS[2]: [7, 7, 6, 11, 12, 5, 6, 9]
}
# 定义遗传算法参数
POPULATION_SIZE = 50
CROSSOVER_PROBABILITY = 0.6
MUTATION_PROBABILITY = 0.01
MAX_GENERATIONS = 100
TOURNAMENT_SIZE = 3
# 定义遗传算法操作
def decode_chromosome(chromosome):
return [HEIGHT_OPTIONS[int(ch)] for ch in chromosome]
def evaluate_fitness(population):
fitness = []
for chromosome in population:
heights = decode_chromosome(chromosome)
oil_consumption = sum([h*c for h,c in zip(heights, OIL_CONSUMPTIONS[START_HEIGHT])])
fitness.append(1.0/oil_consumption)
return np.array(fitness)
def select_parents(population, fitness):
indices = np.random.randint(0, len(population), size=(TOURNAMENT_SIZE, len(population))))
indices_fitness = fitness[indices]
return population[indices[np.argmax(indices_fitness, axis=0), np.arange(len(population))]]
def crossover(parents):
crossover_points = np.random.randint(1, SEGMENTS, size=len(parents))
offspring = np.empty_like(parents)
for i, point in enumerate(crossover_points):
offspring[i] = np.concatenate((parents[i][:point], parents[(i+1)%len(parents)][point:]))
return offspring
def mutate(offspring):
mutation_points = np.random.rand(*offspring.shape) < MUTATION_PROBABILITY
for i, points in enumerate(mutation_points):
offspring[i, points] = np.random.randint(0, len(HEIGHT_OPTIONS), size=points.sum())
return offspring
# 初始化种群
population = np.random.randint(0, len(HEIGHT_OPTIONS), size=(POPULATION_SIZE, SEGMENTS))
# 迭代遗传算法
best_fitness = []
mean_fitness = []
for generation in range(MAX_GENERATIONS):
fitness = evaluate_fitness(population)
best_fitness.append(fitness.max())
mean_fitness.append(fitness.mean())
parents = select_parents(population, fitness)
offspring = crossover(parents)
offspring = mutate(offspring)
population = np.concatenate((population, offspring))
# 绘制适应度值、适应度平均值随迭代次数的变化情况
plt.plot(best_fitness, label='Best fitness')
plt.plot(mean_fitness, label='Mean fitness')
plt.xlabel('Generation')
plt.ylabel('Fitness')
plt.legend()
plt.show()
```
运行这段代码,就可以得到适应度值、适应度平均值随迭代次数的变化情况的图表。
阅读全文
相关推荐
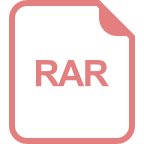
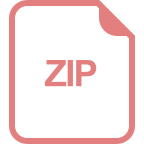
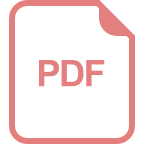

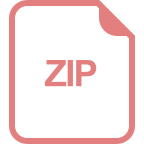
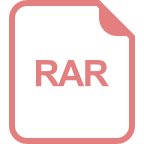
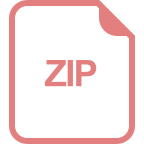
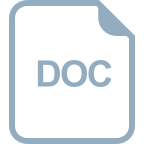
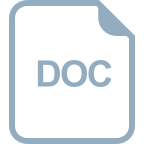
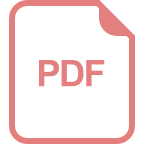
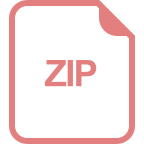
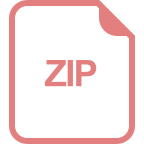
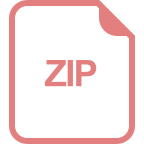
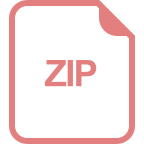
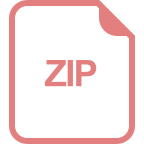