java填充wordxml格式,java xml转成word文档格式
时间: 2024-06-02 20:02:10 浏览: 87
要使用Java填充Word XML格式,可以使用Apache POI库。以下是一个简单的示例:
```java
import java.io.*;
import org.apache.poi.*;
import org.apache.poi.xwpf.usermodel.*;
public class WordXmlFiller {
public static void main(String[] args) {
try {
// Load the Word XML template
FileInputStream fis = new FileInputStream("template.xml");
XWPFDocument document = new XWPFDocument(fis);
// Get the document's body element
XWPFParagraph body = document.createParagraph();
body.setAlignment(ParagraphAlignment.CENTER);
// Create a run of text to insert into the body
XWPFRun run = body.createRun();
run.setText("Hello, World!");
// Save the document to a file
FileOutputStream fos = new FileOutputStream("output.docx");
document.write(fos);
fos.close();
System.out.println("Word document generated successfully!");
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
要将XML转换为Word文档,可以使用JAXB库。以下是一个简单的示例:
```java
import java.io.*;
import javax.xml.bind.*;
import org.docx4j.openpackaging.packages.*;
import org.docx4j.openpackaging.parts.WordprocessingML.*;
public class XmlToWordConverter {
public static void main(String[] args) {
try {
// Load the XML data
JAXBContext jc = JAXBContext.newInstance(Data.class);
Unmarshaller unmarshaller = jc.createUnmarshaller();
Data data = (Data)unmarshaller.unmarshal(new File("data.xml"));
// Load the Word template
WordprocessingMLPackage wordMLPackage = WordprocessingMLPackage.load(new File("template.docx"));
// Get the document's main part
MainDocumentPart documentPart = wordMLPackage.getMainDocumentPart();
// Replace all instances of "{name}" with the name from the data
String name = data.getName();
documentPart.variableReplace("{name}", name);
// Save the document to a file
wordMLPackage.save(new File("output.docx"));
System.out.println("Word document generated successfully!");
} catch (JAXBException e) {
e.printStackTrace();
} catch (Docx4JException e) {
e.printStackTrace();
}
}
}
```
请注意,这些示例可能需要根据您自己的要求进行修改。
阅读全文
相关推荐
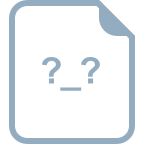
















