Java 将整个文件转SM3值
时间: 2024-12-19 12:27:56 浏览: 23
在Java中,如果你想要将整个文件转换成SM3哈希值,可以使用`java.security.MessageDigest`类配合`javax.crypto.Mac`接口来实现。SM3是一种安全消息摘要算法,类似于MD5或SHA-256。以下是一个简单的步骤:
首先,你需要添加`sm3-core`或类似库,因为Java标准库并不包含SM3。
```java
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.io.File;
import java.nio.charset.StandardCharsets;
import java.security.MessageDigest;
public class FileToSM3 {
public static String fileToSM3Hash(File file) throws Exception {
byte[] bytes = Files.readAllBytes(file.toPath());
SecretKeySpec secretKey = new SecretKeySpec("your_secret_key".getBytes(StandardCharsets.UTF_8), "SM3"); // SM3算法名称
Mac mac = Mac.getInstance("SM3");
mac.init(secretKey);
byte[] hash = mac.doFinal(bytes);
return toHexString(hash); // 转换为十六进制字符串
}
private static String toHexString(byte[] bytes) {
StringBuilder hexString = new StringBuilder();
for (byte b : bytes) {
hexString.append(String.format("%02x", b));
}
return hexString.toString();
}
public static void main(String[] args) {
try {
File file = new File("path_to_your_file");
String sm3Hash = fileToSM3Hash(file);
System.out.println("File's SM3 Hash: " + sm3Hash);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在这个示例中,你需要替换`"your_secret_key"`为你实际使用的SM3密钥。运行这个程序会打印出文件的SM3哈希值。
阅读全文
相关推荐



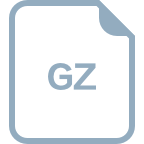














