des加密算法的c++实现
时间: 2023-11-10 08:02:03 浏览: 66
以下是 DES 加密算法的 C 代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/des.h>
int main()
{
// 设置密钥
DES_cblock key;
memset(key, 0, 8);
DES_key_schedule schedule;
DES_set_key_unchecked(&key, &schedule);
// 待加密的明文
const char *plaintext = "Hello World!";
// 加密后的密文
DES_cblock ciphertext;
memset(ciphertext, 0, 8);
DES_ecb_encrypt((const_DES_cblock *)plaintext, &ciphertext, &schedule, DES_ENCRYPT);
// 输出加密结果
printf("Plaintext: %s\n", plaintext);
printf("Ciphertext: ");
int i;
for (i = 0; i < 8; ++i) {
printf("%02X ", ciphertext[i]);
}
printf("\n");
return 0;
}
```
相关问题
DES加密算法C++
DES加密算法(Data Encryption Standard)是一种对称密钥加密算法,其目标是将输入的明文通过DES算法转换成密文。C++中可以使用openssl库来实现DES加密算法。具体步骤如下:
1. 安装openssl库。
2. 导入openssl库的头文件。
3. 生成一个随机的密钥。
4. 使用密钥生成一个初始化向量(IV)。
5. 将明文按照8字节一段进行填充,不足8字节的使用空格填充。
6. 使用DES算法对每一段明文进行加密。
7. 将加密后的密文进行拼接。
8. 将生成的初始化向量和加密后的密文一起输出。
以下是C++代码实现:
```cpp
#include <openssl/des.h>
#include <iostream>
#include <string>
#include <cstring>
using namespace std;
int main()
{
// 生成随机密钥
unsigned char key;
DES_cblock key_block;
DES_random_key(&key_block);
memcpy(key, &key_block, 8);
// 生成初始化向量
unsigned char iv;
DES_cblock iv_block;
memcpy(iv, &iv_block, 8);
// 明文
string plaintext = "hello world";
// 填充明文
int padding = 8 - plaintext.length() % 8;
for (int i = 0; i < padding; i++) {
plaintext += " ";
}
// 加密
string ciphertext;
DES_key_schedule schedule;
DES_set_key(&key_block, &schedule);
for (int i = 0; i < plaintext.length(); i += 8) {
DES_cblock input_block, output_block;
memcpy(input_block, plaintext.c_str() + i, 8);
DES_ncbc_encrypt(&input_block, &output_block, 8, &schedule, &iv_block, DES_ENCRYPT);
ciphertext.append((char*)output_block, 8);
memcpy(iv, output_block, 8);
}
// 输出
cout << "Key: ";
for (int i = 0; i < 8; i++) {
printf("%02x", key[i]);
}
cout << endl;
cout << "IV: ";
for (int i = 0; i < 8; i++) {
printf("%02x", iv[i]);
}
cout << endl;
cout << "Ciphertext: " << ciphertext << endl;
return 0;
}
```
des加密算法c++代码
### 回答1:
DES(Data Encryption Standard)是一种对称加密算法,是密码学中使用最广泛的加密算法之一。下面是使用C语言实现DES加密算法的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/des.h>
// 将16进制字符转换为整数
int hexCharToInt(char c) {
if (c >= '0' && c <= '9') {
return c - '0';
} else if (c >= 'A' && c <= 'F') {
return c - 'A' + 10;
} else if (c >= 'a' && c <= 'f') {
return c - 'a' + 10;
} else {
return -1;
}
}
// 将16进制字符串转换为字节数组
void hexStringToBytes(const char* hexString, unsigned char* bytes) {
int len = strlen(hexString);
int i;
for (i = 0; i < len / 2; i++) {
int high = hexCharToInt(hexString[i * 2]);
int low = hexCharToInt(hexString[i * 2 + 1]);
bytes[i] = (high << 4) + low;
}
}
// DES加密函数
void encryptDES(const unsigned char* key, const unsigned char* plainText, unsigned char* cipherText) {
DES_cblock keyEncrypt;
DES_key_schedule keySchedule;
memcpy(keyEncrypt, key, sizeof(keyEncrypt));
DES_set_odd_parity(&keyEncrypt);
DES_set_key_checked(&keyEncrypt, &keySchedule);
DES_cblock inputText;
memcpy(inputText, plainText, sizeof(inputText));
DES_ecb_encrypt(&inputText, &cipherText, &keySchedule, DES_ENCRYPT);
}
int main() {
const char* keyString = "0123456789ABCDEF";
const char* plainTextString = "Hello World!";
unsigned char key[8];
unsigned char plainText[8];
unsigned char cipherText[8];
hexStringToBytes(keyString, key);
memcpy(plainText, plainTextString, sizeof(plainText));
encryptDES(key, plainText, cipherText);
printf("密钥: %s\n", keyString);
printf("明文: %s\n", plainTextString);
printf("密文: ");
int i;
for (i = 0; i < sizeof(cipherText); i++) {
printf("%02X", cipherText[i]);
}
printf("\n");
return 0;
}
```
以上是一个基于OpenSSL库实现的DES加密算法的C语言代码。代码中包括了将16进制字符串转换为字节数组的函数、DES加密函数以及一个简单的示例在`main`函数中使用这些函数进行加密操作。其中,`keyString`为密钥,`plainTextString`为明文,加密后得到的密文存储在`cipherText`数组中。运行代码可以看到输出的密钥、明文和密文。
### 回答2:
DES(Data Encryption Standard)是一种对称密钥加密算法,使用相同的密钥进行加密和解密。以下是一个简单的使用C语言实现的DES加密算法代码:
```c
#include <stdio.h>
// 初始置换IP矩阵
int IP_table[64] = {58, 50, 42, 34, 26, 18, 10, 2,
60, 52, 44, 36, 28, 20, 12, 4,
62, 54, 46, 38, 30, 22, 14, 6,
64, 56, 48, 40, 32, 24, 16, 8,
57, 49, 41, 33, 25, 17, 9, 1,
59, 51, 43, 35, 27, 19, 11, 3,
61, 53, 45, 37, 29, 21, 13, 5,
63, 55, 47, 39, 31, 23, 15, 7};
// DES加密函数
void des_encrypt(unsigned char *plainText, unsigned char *key, unsigned char *cipherText) {
// 实现DES加密算法的过程
// ...
}
int main() {
unsigned char plainText[8] = "12345678"; // 明文
unsigned char key[8] = "abcdefgh"; // 密钥
unsigned char cipherText[8]; // 加密后的密文
des_encrypt(plainText, key, cipherText); // 加密处理
printf("密文:");
for (int i = 0; i < 8; i++) {
printf("%02X ", cipherText[i]); // 打印密文的十六进制表示
}
printf("\n");
return 0;
}
```
以上代码只是一个简化的示例,实际的DES算法涉及更多的步骤和运算。对于实际应用中的DES加密,建议使用专门的加密库或工具,以确保加密的安全性和正确性。
相关推荐
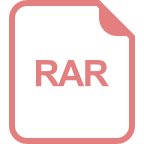
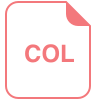
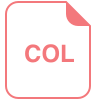
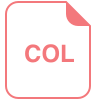









