移动应用开发app代码
时间: 2024-08-13 17:07:47 浏览: 89
移动应用开发通常涉及到两种主要平台:iOS和Android。它们分别使用Objective-C(Swift)和Java(Kotlin)作为主要开发语言。以下是构建一个基本的移动应用的概要:
1. **项目设置**:
- iOS: 使用Xcode创建新项目,选择Swift或Objective-C作为开发语言。
- Android: 在Android Studio中创建新项目,选择Java或Kotlin。
2. **界面设计**:
- 使用UI框架,如UIKit (iOS) 或 Android SDK中的Material Design(Android),设计用户界面。
3. **数据模型**:
- 创建数据模型类,描述应用的数据结构,如用户、商品等。
4. **功能实现**:
- 使用网络请求库(如Alamofire, URLSession for iOS,OkHttp for Android)处理数据交互。
- 实现业务逻辑,如登录、注册、推送通知等。
5. **事件驱动**:
- 用MVVM(Model-View-ViewModel)或 MVP(Model-View-Presenter)架构模式组织代码,让视图层独立于业务逻辑。
6. **用户体验**:
- 优化应用程序性能,考虑用户体验(动画、缓存、错误处理等)。
7. **测试**:
- 编写单元测试和集成测试确保功能正常。
8. **发布**:
- 提交到App Store或Google Play Store进行审核和发布。
阅读全文
相关推荐
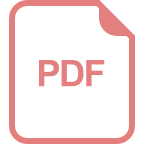
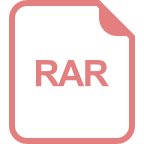
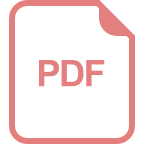
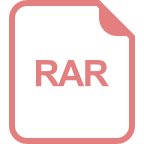
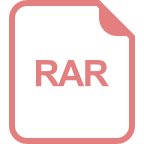
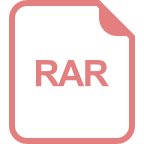
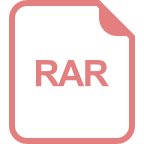
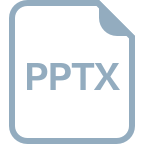
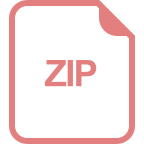
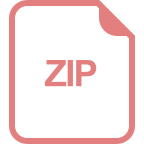
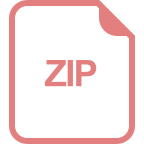
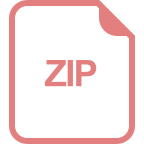
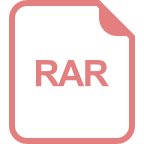
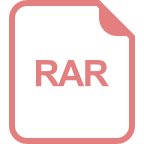
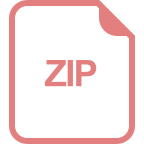
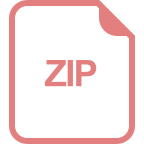
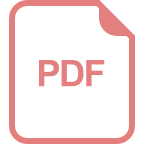
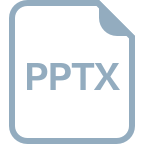
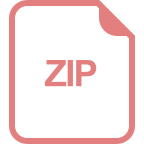