python基于opencv人脸识别源码
时间: 2024-12-25 15:26:15 浏览: 0
在Python中使用OpenCV进行人脸识别通常涉及以下几个步骤:
1. **安装库**:首先需要安装`opencv-python`和`face_recognition`库。可以使用pip命令进行安装:
```
pip install opencv-python face_recognition
```
2. **加载预训练模型**:OpenCV本身并不包含人脸识别的内置算法,但你可以使用它作为面部识别API的底层框架。比如dlib库提供的人脸检测器,然后结合`face_recognition`库的特征提取功能。
```python
import cv2
from imutils import face_utils
import dlib
import face_recognition
# 加载人脸检测器
detector = dlib.get_frontal_face_detector()
```
3. **捕获图像或视频流**:
```python
cap = cv2.VideoCapture('path_to_your_video.mp4')
while True:
# 读取帧
ret, frame = cap.read()
if not ret:
break
```
4. **人脸检测**:
```python
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 使用dlib检测人脸
faces = detector(gray)
```
5. **人脸识别**:
```python
known_faces = []
# 假设你知道一些已知人脸的编码
for filename in ['known_face1.jpg', 'known_face2.jpg']:
img = face_recognition.load_image_file(filename)
encoding = face_recognition.face_encodings(img)[0]
known_faces.append(encoding)
for face in faces:
# 提取当前帧的面部特征
face_encoding = face_recognition.face_encodings(face)[0]
# 比较特征并找出最相似的脸
results = face_recognition.compare_faces(known_faces, face_encoding)
match = results.index(True) if results else None
# 根据匹配结果画框或做其他处理
...
```
6. **可视化结果**:
找到匹配的人脸后,可以在图像上绘制边框或显示标签。
记得在实际应用中,你可能需要对上述步骤进行适当的调整,并处理可能出现的各种异常情况。如果你对某个部分有更深入的需求,如自定义训练人脸识别模型,那么还需要学习深度学习的知识。
阅读全文
相关推荐
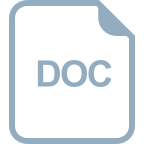
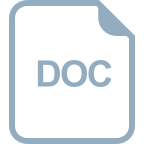
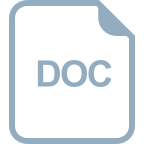
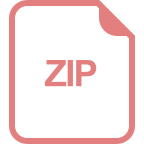
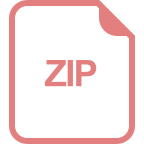
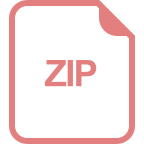
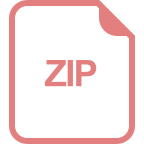
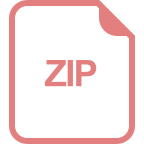
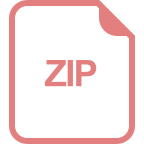
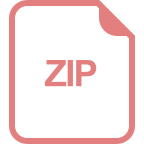
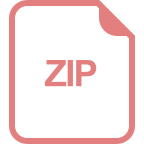
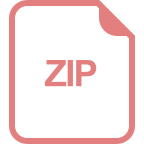
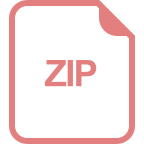
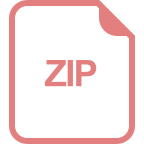
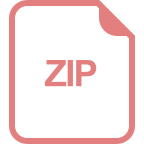
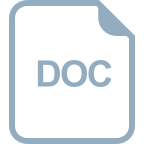
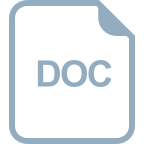
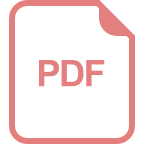
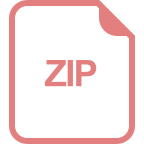