C#WPF用户控件界面中的图片根据我输入的像素坐标xy和像素宽高,在图片上自动画出该区域的框,详细代码展示
时间: 2024-12-18 07:15:02 浏览: 12
在C# WPF中,如果你想要根据用户输入的像素坐标和大小绘制一个矩形框在图片上,你可以利用`Rectangle`元素以及一些基本的数学计算。以下是一个简单的示例代码,它创建了一个`UserControl`并实现了一个方法用于绘制矩形:
```csharp
using System.Windows;
using System.Windows.Controls;
using System.Windows.Media;
public partial class MyImageControl : UserControl
{
private Image _imageControl;
public static readonly DependencyProperty PixelCoordinatesProperty = DependencyProperty.Register("PixelCoordinates", typeof(Point), typeof(MyImageControl), new FrameworkPropertyMetadata(default(Point)));
public static readonly DependencyProperty SizeInPixelsProperty = DependencyProperty.Register("SizeInPixels", typeof(Size), typeof(MyImageControl), new FrameworkPropertyMetadata(default(Size)));
public Point PixelCoordinates
{
get { return (Point)GetValue(PixelCoordinatesProperty); }
set { SetValue(PixelCoordinatesProperty, value); UpdateDrawing(); }
}
public Size SizeInPixels
{
get { return (Size)GetValue(SizeInPixelsProperty); }
set { SetValue(SizeInPixelsProperty, value); UpdateDrawing(); }
}
public MyImageControl()
{
InitializeComponent();
_imageControl = this.FindName("MyImage") as Image;
if (_imageControl != null)
{
_imageControl.Loaded += OnLoaded;
}
}
private void OnLoaded(object sender, RoutedEventArgs e)
{
UpdateDrawing();
}
private void UpdateDrawing()
{
if (_imageControl != null && PixelCoordinates != default(Point) && SizeInPixels != default(Size))
{
var drawingVisual = new DrawingVisual();
using (var context = drawingVisual.RenderOpen())
{
var pen = new Pen(Brushes.Black, 2);
context.DrawRectangle(pen, null, new Rect(new Point(PixelCoordinates.X, PixelCoordinates.Y), SizeInPixels));
}
_imageControl.Source = drawingVisual.ToBitmapSource();
}
}
}
// 使用这个控件的方式:
<my:MyImageControl PixelCoordinates="10,10" SizeInPixels="50,50">
<my:MyImageControl.MyImage Stretch="Uniform" Source="path_to_your_image.jpg"/>
</my:MyImageControl>
```
在这个例子中,你需要设置`PixelCoordinates`属性为左上角的坐标(x, y),`SizeInPixels`属性为矩形的宽度和高度。当图像加载完成时,会根据这些值在图像上绘制一个黑色边框。
阅读全文
相关推荐
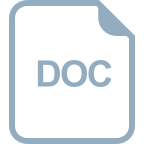
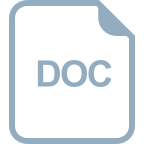
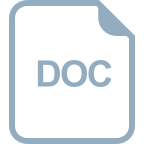


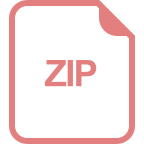
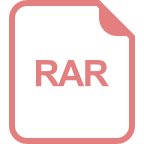
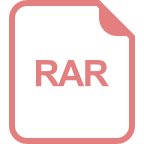
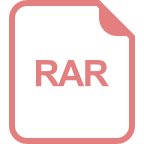
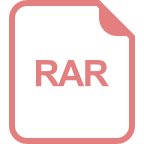
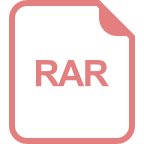


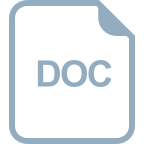
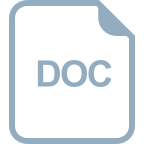
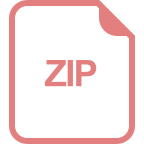
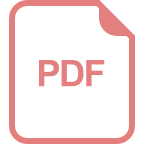
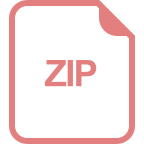