c语言读入文件,并且存储里面的字符
时间: 2024-11-06 18:16:00 浏览: 12
在C语言中,读取文件并存储其内容通常涉及到文件操作的基本概念,如`fopen()`, `fclose()`, `fread()`等函数。下面是一个简单的示例,演示如何打开、读取文本文件并将字符存入内存:
```c
#include <stdio.h> // 包含文件操作的头文件
int main() {
FILE *file; // 定义文件指针
char content[100]; // 存储文件内容的数组
size_t bytesRead;
// 打开文件,如果失败则返回错误
if ((file = fopen("example.txt", "r")) == NULL) {
printf("Failed to open file.\n");
return 1;
}
// 试图从文件中读取数据,最多读取99个字节,防止溢出
bytesRead = fread(content, sizeof(char), 100, file);
// 检查是否成功读取
if (bytesRead == 0) {
printf("Reading from the file failed.\n");
fclose(file); // 关闭文件
return 1;
} else if (feof(file)) { // 判断文件结束
printf("End of file reached.\n");
} else {
// 文件已成功读取,关闭文件
fclose(file);
// 输出读取的内容
printf("Content read: %s\n", content);
}
return 0;
}
```
在这个例子中,我们假设文件名为"example.txt"。程序会尝试打开这个文件,然后读取其中的内容到`content`数组中。最后关闭文件并显示读取的结果。
阅读全文
相关推荐
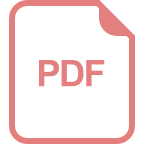
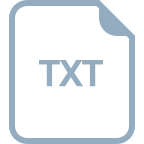
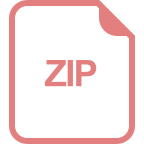















