Python 读取命令行参数
时间: 2024-08-16 19:04:18 浏览: 111
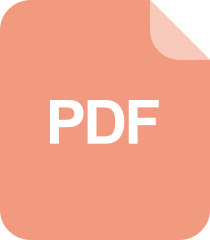
python实现读取命令行参数的方法
在Python中,你可以通过`sys`模块来读取命令行传递给程序的参数。当你运行Python脚本时,可以像这样指定额外的参数:
```python
import sys
# 获取命令行参数列表
args = sys.argv
# 第一个元素是脚本名称,从索引1开始获取实际参数
first_arg = args
second_arg = args if len(args) > 2 else None
print("First argument:", first_arg)
print("Second argument (if provided):", second_arg)
#
阅读全文
相关推荐
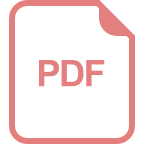
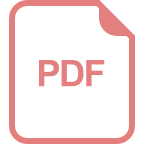

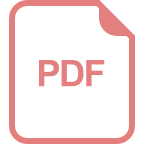
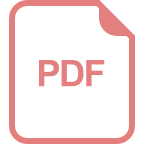
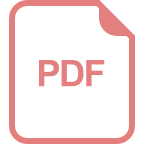

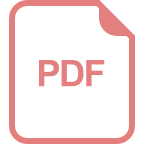
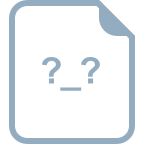
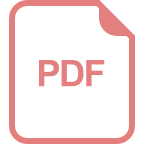
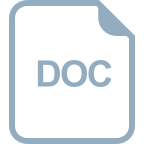
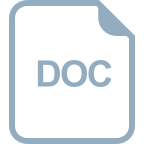
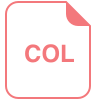
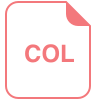


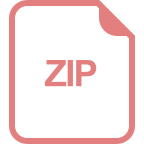
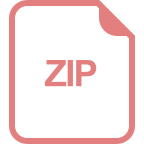
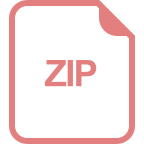