我使用HTML和CSS画了一个象棋的棋盘以及棋子,我该怎么做可以让棋子动起来
时间: 2024-02-06 21:09:16 浏览: 175
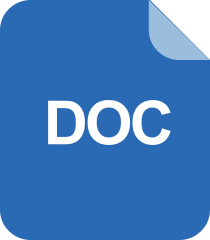
算法与数据结构课程设计:棋盘覆盖实现(使用HTML,CSS,JS)

要让棋子动起来,你需要使用JavaScript来添加交互性。以下是一些实现的步骤:
1. 为每个棋子创建一个JavaScript对象,其中包含其位置、颜色和其他相关信息。
2. 使用JavaScript监听鼠标事件,例如mousedown和mouseup,以便在拖动棋子时更新其位置。
3. 当棋子移动时,使用CSS的transform属性来更新其位置。例如,你可以使用translateX和translateY来移动棋子。
4. 如果需要,可以使用CSS的transition属性来为棋子添加动画效果。
下面是一个简单的示例代码,可以帮助你开始实现棋子的移动:
```html
<!DOCTYPE html>
<html>
<head>
<title>Chess Board</title>
<style>
.chessboard {
display: flex;
flex-wrap: wrap;
width: 400px;
height: 400px;
margin: 0 auto;
}
.square {
box-sizing: border-box;
width: 50px;
height: 50px;
border: 1px solid black;
background-color: #EFEFEF;
}
.piece {
position: absolute;
width: 50px;
height: 50px;
background-image: url("pieces.png");
background-size: 600px 200px;
}
</style>
</head>
<body>
<div class="chessboard">
<div class="square"></div>
<div class="square"></div>
<!-- more squares... -->
</div>
<script>
// Define chess pieces as JavaScript objects
var pieces = [
{ type: "rook", color: "white", position: "a1" },
{ type: "knight", color: "white", position: "b1" },
// more pieces...
];
// Create HTML elements for each piece
pieces.forEach(function(piece) {
var element = document.createElement("div");
element.className = "piece " + piece.type + " " + piece.color;
element.style.backgroundPosition = getBackgroundPosition(piece.type, piece.color);
element.style.left = getLeftOffset(piece.position);
element.style.top = getTopOffset(piece.position);
document.body.appendChild(element);
});
// Define event listeners for mouse events
var draggedPiece = null;
document.addEventListener("mousedown", function(event) {
if (event.target.classList.contains("piece")) {
draggedPiece = event.target;
}
});
document.addEventListener("mouseup", function(event) {
if (draggedPiece) {
draggedPiece.style.transform = "translate(" + event.clientX + "px, " + event.clientY + "px)";
draggedPiece = null;
}
});
// Helper functions to calculate background position and element offset
function getBackgroundPosition(type, color) {
var x = {
"rook": 0,
"knight": -50,
// more positions...
}[type];
var y = {
"white": 0,
"black": -50,
}[color];
return x + "px " + y + "px";
}
function getLeftOffset(position) {
return (position.charCodeAt(0) - "a".charCodeAt(0)) * 50 + "px";
}
function getTopOffset(position) {
return (8 - parseInt(position.charAt(1))) * 50 + "px";
}
</script>
</body>
</html>
```
请注意,这只是一个简单的例子,你需要根据自己的需求进行修改和扩展。
阅读全文
相关推荐
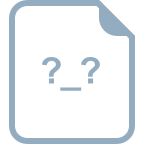
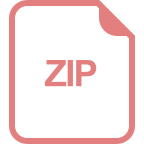
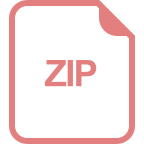
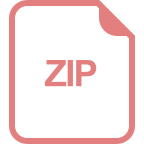
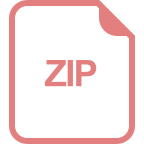
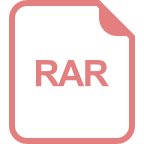
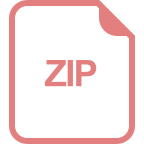
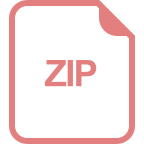
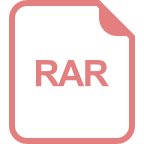
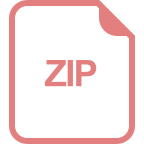
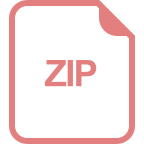
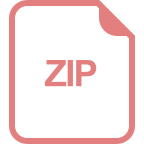
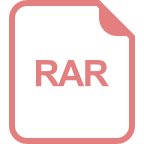
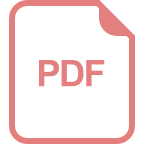
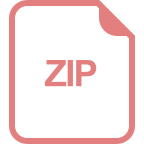
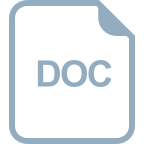