使用Android Studio等工具编写前端代码,实现隐藏密码登录,登陆后的中心框架使用选项卡的形式设计,并且包含音乐、好友、游记和图册四大模块
时间: 2024-02-25 19:57:13 浏览: 25
好的,以下是实现隐藏密码登录、选项卡中心框架的代码示例:
XML布局文件:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<EditText
android:id="@+id/et_username"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="用户名" />
<EditText
android:id="@+id/et_password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="密码"
android:inputType="textPassword" />
<Button
android:id="@+id/btn_login"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="登录" />
<TextView
android:id="@+id/tv_tab_music"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="音乐" />
<TextView
android:id="@+id/tv_tab_friends"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="好友" />
<TextView
android:id="@+id/tv_tab_travel"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="游记" />
<TextView
android:id="@+id/tv_tab_gallery"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="图册" />
<FrameLayout
android:id="@+id/fl_content"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1" />
</LinearLayout>
```
Java代码:
```java
public class MainActivity extends AppCompatActivity {
private EditText etUsername;
private EditText etPassword;
private Button btnLogin;
private TextView tvTabMusic;
private TextView tvTabFriends;
private TextView tvTabTravel;
private TextView tvTabGallery;
private FrameLayout flContent;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
etUsername = findViewById(R.id.et_username);
etPassword = findViewById(R.id.et_password);
btnLogin = findViewById(R.id.btn_login);
tvTabMusic = findViewById(R.id.tv_tab_music);
tvTabFriends = findViewById(R.id.tv_tab_friends);
tvTabTravel = findViewById(R.id.tv_tab_travel);
tvTabGallery = findViewById(R.id.tv_tab_gallery);
flContent = findViewById(R.id.fl_content);
// 隐藏密码登录
btnLogin.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String username = etUsername.getText().toString();
String password = etPassword.getText().toString();
// TODO: 验证用户名和密码是否正确
}
});
// 中心框架使用选项卡的形式设计
tvTabMusic.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO: 切换到音乐模块
showFragment(new MusicFragment());
}
});
tvTabFriends.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO: 切换到好友模块
showFragment(new FriendsFragment());
}
});
tvTabTravel.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO: 切换到游记模块
showFragment(new TravelFragment());
}
});
tvTabGallery.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO: 切换到图册模块
showFragment(new GalleryFragment());
}
});
}
private void showFragment(Fragment fragment) {
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction transaction = fragmentManager.beginTransaction();
transaction.replace(R.id.fl_content, fragment);
transaction.commit();
}
}
```
以上代码实现了隐藏密码登录和选项卡中心框架的设计,其中音乐、好友、游记和图册四大模块需要自行实现对应的Fragment。
相关推荐
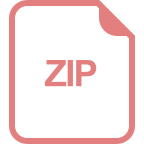
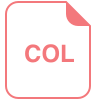
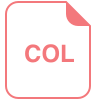
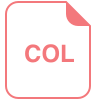
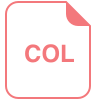
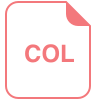









