Bucket Brigade FIFO SRL16E ( VHDL )
时间: 2023-08-08 17:12:45 浏览: 119
The Bucket Brigade FIFO SRL16E is a type of shift register that is commonly used in digital circuits to implement a First-In-First-Out (FIFO) buffer. It operates by shifting data through a series of stages or "buckets", with each stage containing one or more flip-flops. The SRL16E is a specific type of shift register that can store up to 16 bits of data.
In VHDL, the SRL16E can be implemented using a combination of behavioral and structural modeling techniques. The behavioral model describes the functional behavior of the FIFO, while the structural model describes how the various components are interconnected.
Here is an example VHDL code for implementing a Bucket Brigade FIFO SRL16E:
```vhdl
library ieee;
use ieee.std_logic_1164.all;
entity bb_fifo is
port (
clk : in std_logic;
reset : in std_logic;
data_in : in std_logic_vector(15 downto 0);
data_out: out std_logic_vector(15 downto 0);
empty : out std_logic;
full : out std_logic
);
end entity;
architecture rtl of bb_fifo is
signal stage0, stage1, stage2, stage3, stage4, stage5, stage6, stage7,
stage8, stage9, stage10, stage11, stage12, stage13, stage14, stage15 : std_logic_vector(1 downto 0);
begin
-- behavioral model
process(clk, reset)
begin
if reset = '1' then
-- clear all stages
stage0 <= (others => '0');
stage1 <= (others => '0');
stage2 <= (others => '0');
stage3 <= (others => '0');
stage4 <= (others => '0');
stage5 <= (others => '0');
stage6 <= (others => '0');
stage7 <= (others => '0');
stage8 <= (others => '0');
stage9 <= (others => '0');
stage10 <= (others => '0');
stage11 <= (others => '0');
stage12 <= (others => '0');
stage13 <= (others => '0');
stage14 <= (others => '0');
stage15 <= (others => '0');
elsif rising_edge(clk) then
-- shift data through stages
stage0 <= data_in(0) & stage0(1);
stage1 <= stage0(0) & stage1(1);
stage2 <= stage1(0) & stage2(1);
stage3 <= stage2(0) & stage3(1);
stage4 <= stage3(0) & stage4(1);
stage5 <= stage4(0) & stage5(1);
stage6 <= stage5(0) & stage6(1);
stage7 <= stage6(0) & stage7(1);
stage8 <= stage7(0) & stage8(1);
stage9 <= stage8(0) & stage9(1);
stage10 <= stage9(0) & stage10(1);
stage11 <= stage10(0) & stage11(1);
stage12 <= stage11(0) & stage12(1);
stage13 <= stage12(0) & stage13(1);
stage14 <= stage13(0) & stage14(1);
stage15 <= stage14(0) & stage15(1);
end if;
end process;
-- structural model
data_out <= stage15 & stage14 & stage13 & stage12 & stage11 & stage10 & stage9 & stage8 &
stage7 & stage6 & stage5 & stage4 & stage3 & stage2 & stage1 & stage0;
empty <= stage15(0);
full <= stage0(1);
end architecture;
```
This VHDL code implements a 16-bit Bucket Brigade FIFO SRL16E with a behavioral model for shifting data through the stages and a structural model for connecting the stages and outputting the data. It also includes inputs for clock and reset signals, as well as outputs for the data_out, empty, and full signals.
阅读全文
相关推荐


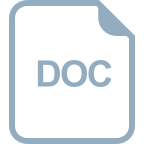














