用vue写出一个按钮匹配一个勾选框,写四行。第一行延时0.1秒触发。第二行0.2秒依次类推。然后有个开始按钮。勾选后按钮后。比如勾选了第一行。第三行。分别在每0.1秒点击下第一行按钮。每0.3秒点击下第三行按钮,并增加点击动画。开始按钮点击后变成停止按钮并变色
时间: 2023-08-23 17:04:53 浏览: 42
以下是用 Vue 实现的代码:
```html
<template>
<div>
<div v-for="(item, index) in items" :key="index">
<input type="checkbox" v-model="checked[index]">
<button :class="{ active: active[index] }" @click="clickButton(index)">{{ index + 1 }}</button>
</div>
<button :class="{ active: isRunning }" @click="toggleRun">{{ isRunning ? '停止' : '开始' }}</button>
</div>
</template>
<script>
export default {
data() {
return {
items: [0.1, 0.2, 0.3, 0.4],
checked: [false, false, false, false],
active: [false, false, false, false],
isRunning: false,
timer: null
}
},
methods: {
clickButton(index) {
if (this.checked[index]) {
this.active[index] = true
setTimeout(() => {
this.active[index] = false
}, 300)
}
},
toggleRun() {
if (this.isRunning) {
clearInterval(this.timer)
this.isRunning = false
} else {
let currentIndex = 0
this.timer = setInterval(() => {
if (this.checked[currentIndex]) {
this.clickButton(currentIndex)
}
currentIndex = (currentIndex + 1) % this.items.length
}, 100 * (currentIndex + 1))
this.isRunning = true
}
}
}
}
</script>
<style>
button {
margin-right: 10px;
padding: 5px;
border: none;
border-radius: 4px;
background-color: #ccc;
transition: all 0.3s ease;
}
button.active {
background-color: #f00;
color: #fff;
box-shadow: 0 0 10px #f00;
}
button:last-child {
margin-right: 0;
}
</style>
```
实现思路如下:
- 通过 `v-for` 指令遍历 `items` 数组,生成多个勾选框和按钮;
- 给每个勾选框绑定 `v-model`,双向绑定到 `checked` 数组中;
- 给每个按钮绑定 `:class` 和 `@click`,根据 `active` 数组控制按钮样式,根据勾选框选中状态决定是否触发点击事件;
- 给开始/停止按钮绑定 `:class` 和 `@click`,根据 `isRunning` 变量状态控制按钮样式和定时器的启停。
具体实现中,定时器的回调函数中,首先判断当前勾选框是否选中,如果是,则触发对应的按钮点击事件,然后将当前索引加 1,如果超出了 `items` 数组的长度,则将索引重置为 0。按钮点击事件中,通过 `setTimeout` 函数实现点击动画效果。
相关推荐
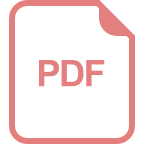



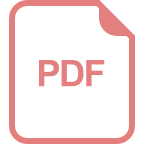
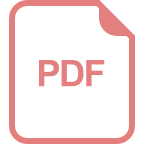
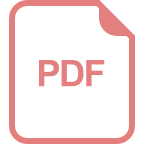
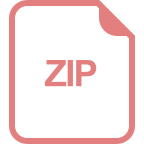
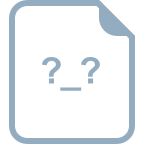
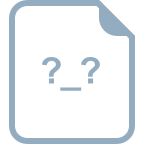
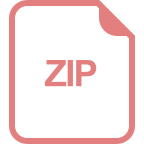
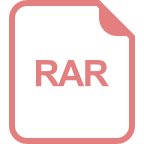
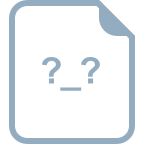