java和geoserver发布geojson格式的矢量切片
时间: 2024-06-09 17:06:28 浏览: 124
您可以使用Java编写一个程序,使用GeoTools库来读取您的矢量数据,并使用GeoJSON格式将其转换为瓦片。然后,您可以使用GeoServer来发布这些瓦片。
下面是一个简单的Java程序,可以将矢量数据转换为GeoJSON格式的瓦片:
```java
import java.io.File;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import org.geotools.data.simple.SimpleFeatureCollection;
import org.geotools.data.simple.SimpleFeatureIterator;
import org.geotools.data.store.ContentFeatureCollection;
import org.geotools.factory.CommonFactoryFinder;
import org.geotools.feature.FeatureIterator;
import org.geotools.feature.simple.SimpleFeatureBuilder;
import org.geotools.feature.simple.SimpleFeatureTypeBuilder;
import org.geotools.geojson.feature.FeatureJSON;
import org.geotools.geometry.jts.JTSFactoryFinder;
import org.geotools.map.FeatureLayer;
import org.geotools.map.MapContent;
import org.geotools.referencing.crs.DefaultGeographicCRS;
import org.geotools.renderer.lite.StreamingRenderer;
import org.geotools.styling.SLD;
import org.geotools.styling.Style;
import org.geotools.styling.StyleFactory;
import org.geotools.styling.Symbolizer;
import org.geotools.styling.TextSymbolizer;
import org.geotools.styling.Font;
import org.locationtech.jts.geom.Envelope;
import org.locationtech.jts.geom.Geometry;
import org.locationtech.jts.geom.Point;
import org.locationtech.jts.geom.Polygon;
import org.opengis.feature.simple.SimpleFeature;
import org.opengis.feature.simple.SimpleFeatureType;
import org.opengis.filter.FilterFactory2;
import org.opengis.filter.identity.FeatureId;
public class GeoJSONTileGenerator {
public static void main(String[] args) throws IOException {
// Open the vector data file
File file = new File("data.shp");
Map<String, Object> map = new HashMap<>();
map.put("url", file.toURI().toURL());
// Read the data into a feature collection
ContentFeatureCollection features = new ContentFeatureCollection(map, null);
// Create a style for the features
StyleFactory styleFactory = CommonFactoryFinder.getStyleFactory();
FilterFactory2 filterFactory = CommonFactoryFinder.getFilterFactory2();
Symbolizer symbolizer = styleFactory.createPolygonSymbolizer();
Style style = SLD.createPolygonStyle(java.awt.Color.BLUE, java.awt.Color.WHITE, 1.0f);
TextSymbolizer textSymbolizer = styleFactory.createTextSymbolizer();
Font font = styleFactory.getDefaultFont();
textSymbolizer.setFont(font);
textSymbolizer.setLabel("Name");
style.featureTypeStyles().get(0).rules().get(0).symbolizers().add(textSymbolizer);
// Create a feature type for the GeoJSON output
SimpleFeatureTypeBuilder builder = new SimpleFeatureTypeBuilder();
builder.setName("MyFeatureType");
builder.setCRS(DefaultGeographicCRS.WGS84);
builder.add("geometry", Geometry.class);
builder.add("Name", String.class);
SimpleFeatureType featureType = builder.buildFeatureType();
// Create a GeoJSON feature writer
FeatureJSON featureJSON = new FeatureJSON();
featureJSON.setEncodeNullValues(true);
// Create a map content for the renderer
MapContent mapContent = new MapContent();
mapContent.setTitle("My Map");
// Add the features to the map content and set the style
SimpleFeatureIterator iterator = features.features();
while (iterator.hasNext()) {
SimpleFeature feature = iterator.next();
FeatureId id = feature.getIdentifier();
Envelope envelope = feature.getBounds();
Geometry geometry = (Geometry) feature.getDefaultGeometry();
SimpleFeatureBuilder featureBuilder = new SimpleFeatureBuilder(featureType);
featureBuilder.add(geometry);
featureBuilder.add(feature.getAttribute("Name"));
SimpleFeature newFeature = featureBuilder.buildFeature(id.toString());
mapContent.addLayer(new FeatureLayer(newFeature, style));
}
iterator.close();
// Create a renderer for the map content and set the bounding box
StreamingRenderer renderer = new StreamingRenderer();
renderer.setMapContent(mapContent);
renderer.setJava2DHints(new java.util.HashMap<RenderingHints.Key,Object>());
Envelope bbox = new Envelope(-180, 180, -90, 90);
double resolution = 0.1;
int tileSize = 256;
// Loop through the tiles and write them to GeoJSON files
for (int x = 0; x < Math.pow(2, 5); x++) {
for (int y = 0; y < Math.pow(2, 5); y++) {
Envelope tileEnvelope = new Envelope(x * tileSize * resolution + bbox.getMinX(),
(x + 1) * tileSize * resolution + bbox.getMinX(),
y * tileSize * resolution + bbox.getMinY(),
(y + 1) * tileSize * resolution + bbox.getMinY());
renderer.setMapContent(mapContent);
renderer.paint(null, null, tileEnvelope);
Geometry tileGeometry = JTSFactoryFinder.getGeometryFactory().toGeometry(tileEnvelope);
SimpleFeatureBuilder featureBuilder = new SimpleFeatureBuilder(featureType);
featureBuilder.add(tileGeometry);
featureBuilder.add(null);
SimpleFeature feature = featureBuilder.buildFeature(null);
File tileFile = new File("tile_" + x + "_" + y + ".json");
featureJSON.writeFeature(feature, tileFile);
}
}
}
}
```
这个程序将矢量数据读取到一个特征集合中,然后为每个特征创建一个图层,并使用GeoTools渲染器将其渲染为一个GeoJSON格式的瓦片。您可以根据需要修改渲染器的参数,例如分辨率和瓦片大小。
然后,您可以使用GeoServer来发布这些瓦片。首先,将它们上传到GeoServer中。然后,创建一个新的数据存储,选择“GeoJSON瓦片”作为存储类型,并选择适当的参数。最后,发布存储并选择适当的样式。
阅读全文
相关推荐
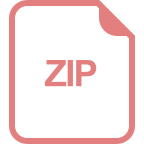
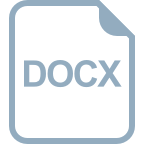
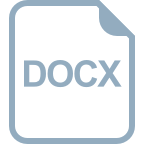
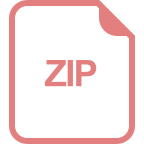
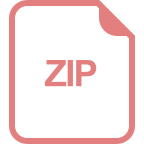
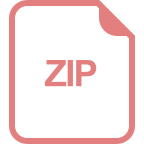
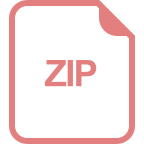
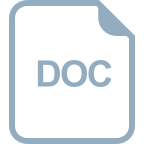
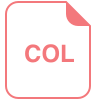

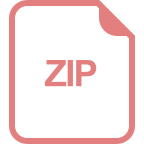
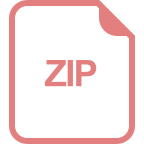