obs为什么找不到mp3解码器
时间: 2023-10-04 10:07:14 浏览: 313
OBS(Open Broadcaster Software)找不到 MP3 解码器可能是因为您的计算机上缺少相关的解码器。您可以尝试安装 LAME MP3 解码器(Windows 平台上可用),或 FFmpeg 解码器库(跨平台)。如果您已经安装了这些解码器,那么可能是您的 OBS 配置有误,您可以检查设置中的音频选项以确保它们正确配置。如果问题仍然存在,您可以尝试卸载并重新安装 OBS。
相关问题
写一段python代码实现用指针网络深度强化学习AC算法解决三维TSP避障问题,两点之间的距离使用欧氏距离,其中P(x,y,z)点为旅行商要经过的城市点,数量不固定,O(x,y,z)是障碍点,数量也不固定,路径遇到障碍点要避开,最后明确目标函数以及优化函数,奖励函数
在Python中,实现基于指针网络(Pointer Network)的深度强化学习算法(如Actor-Critic)来解决三维TSP避障问题是一个复杂的任务,因为这涉及到深度学习模型、环境交互和强化学习策略。下面是一个简化的例子,展示了如何构建一个基本框架,但我们需要知道的是,实际部署这样的算法需要大量的数据预处理、模型训练和高级优化技术。
首先,我们需要导入所需的库:
```python
import numpy as np
from collections import defaultdict
import gym
from ray.rllib.agents.ppo import PPOTrainer
from ray.rllib.models.tf.fcnet_v2 import FullyConnectedNetwork
from ray.rllib.models.tf.torch_modelv2 import TorchModelV2
```
然后定义一个简单的环境类(这里仅作示例,实际环境中需要更复杂的设计):
```python
class TSPEnv(gym.Env):
def __init__(self, cities, obstacles):
self.cities = cities
self.obstacles = obstacles
self.action_space = gym.spaces.Discrete(len(cities) - 1)
self.observation_space = gym.spaces.Dict(
{
"city": gym.spaces.Box(low=0, high=len(self.cities) - 1),
"obstacle_map": gym.spaces.Box(low=-np.inf, high=np.inf, shape=(len(self.obstacles), len(self.obstacles))),
}
)
# ... implement step() and reset() methods here
```
接下来,定义一个使用指针网络的模型:
```python
class PointerNetModel(TorchModelV2):
def __init__(self, obs_space, action_space, num_outputs, model_config, name):
super().__init__(obs_space, action_space, num_outputs, model_config, name)
# ... define your network architecture with pointers for city selection
```
现在我们可以设置`ray`环境并创建强化学习训练器:
```python
def create_agent(env, config):
config["model"] = {"custom_model": PointerNetModel}
config["num_workers"] = 0 # For simplicity, use a single worker in this example
trainer = PPOTrainer(config=config, env=env)
return trainer
# Create environment and train the agent
env = TSPEnv(..., ...)
trainer = create_agent(env, ...)
# Define reward function (negative distance to closest obstacle + penalty for collisions)
def reward_function(obs, next_obs, action, **kwargs):
# ... calculate reward based on distance from obstacles and penalties
# Train the agent with actor-critic algorithm, using reward_function
for _ in range(num_episodes):
done = False
obs = env.reset()
while not done:
action = trainer.compute_action(obs)
next_obs, reward, done, _ = env.step(action)
trainer.train_batch({"obs": obs, "actions": action, "rewards": [reward]})
obs = next_obs
# Update target networks periodically
trainer.update_target()
```
注意这个代码只是一个基础框架,实际应用中需要处理更多的细节,比如状态空间转换、动态规划解码等。此外,对于三维TSP,通常会使用特殊的编码和解码技巧,以及考虑空间维度带来的计算挑战。
阅读全文
相关推荐
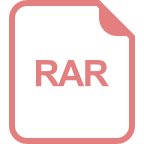
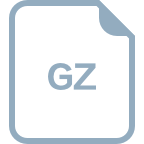
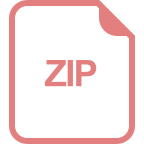
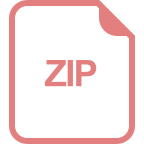
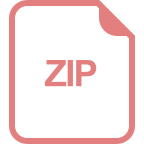
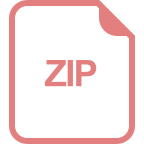
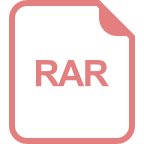
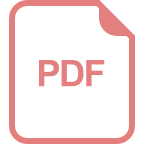
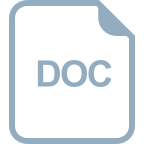
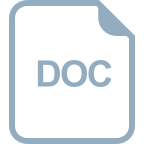
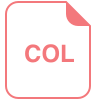
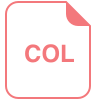
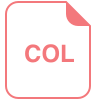
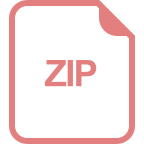
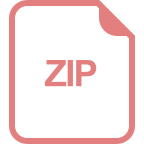
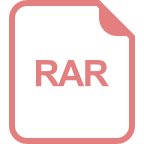
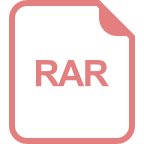