function Save(){ var username; httpRequest=new XMLHttpRequest(); httpRequest.open("POST","Home",false); httpRequest.send(); httpRequest.onreadystatechange=function (){ if (httpRequest.readyState==4&&httpRequest.status==200){ username=httpRequest.response; } } alert(username); } 代码alert为什么弹出undefined
时间: 2024-03-17 20:47:06 浏览: 90
代码中`alert(username);`弹出`undefined`的原因是因为Ajax请求是异步的,而该代码使用的是同步方式发送请求,所以在`alert(username);`执行时,`httpRequest.onreadystatechange`回调函数还未执行,`username`变量没有被赋值,因此`alert`弹出的是`undefined`。
要获取正确的`username`值,可以将`alert(username);`语句放到`httpRequest.onreadystatechange`回调函数内部,如下所示:
```javascript
function Save() {
var username;
httpRequest = new XMLHttpRequest();
httpRequest.open("POST", "Home", false);
httpRequest.send();
httpRequest.onreadystatechange = function() {
if (httpRequest.readyState == 4 && httpRequest.status == 200) {
username = httpRequest.response;
alert(username); // 在回调函数内部弹出正确的结果
}
}
}
```
这样就可以正确获取到`username`变量的值了。
相关问题
<script type="text/javascript"> var httpRequest = new XMLHttpRequest(); httpRequest.open('GET','http://1.14.137.211:8888/tealist',true); httpRequest.send(); httpRequest.onreadystatechange = function(){ if(httpRequest.readyState == 4 && httpRequest.status ==200 ){ var data = httpRequest.responseText; var data = JSON.parse(data); console.log(data); function test1(){ $(".increment").onclick = function(){ let n = $(this).siblings(".itxt").val(); console.log(n); n++; $(this).siblings(".itxt").val(n); } return; } function test2(){ $(".decrement").onclick = function(){ let n = $(this).siblings(".itxt").val(); console.log(n); if (n == 0) { return false; } n--; $(this).siblings(".itxt").val(n); } return; } data.forEach(element => { let html = ` <p> <span>${element.name}</span><span class="price">${element.price}¥</span> <span><a class="decrement" onclick = ${test1()}>-</a> <input type="text" class="itxt" value="0"> <a class="increment" onclick = ${test2()}>+</a></span> </p> ` $("#pics").append(html); //document.querySelector("#pics").innerHTML = html; }); } } </script>
这段代码是一个 AJAX 请求,它向指定的 URL 发送一个 GET 请求,获取返回的数据并用 JSON 解析。然后它将这些数据添加到 HTML 页面中,动态地生成一组商品列表。
具体来说,这段代码实现了以下功能:
1. 创建一个 XMLHttpRequest 对象。
2. 使用 open() 方法指定请求的 URL、请求方式和是否异步方式发送请求。
3. 使用 send() 方法发送请求。
4. 使用 onreadystatechange 事件监听器,当 readyState 属性值为 4(请求已完成)且 status 属性为 200(请求成功)时,解析返回的数据并将其添加到页面中。
5. 在数据添加到页面中时,动态生成一组商品列表,每个商品包含名称、价格和数量输入框。
6. 给每个数量输入框增加了两个按钮,分别用于增加和减少数量,并且在点击按钮时会触发相应的事件处理函数 test1() 和 test2()。
需要注意的是,test1() 和 test2() 函数中的代码似乎有问题,因为它们使用了 $() 函数,但是该函数没有在代码中定义。可能是使用了某个前端框架或库,需要查看完整代码才能确定。
js代码中httpRequest介绍
httpRequest是一个封装了XMLHttpRequest对象的JavaScript函数,用于在Web应用程序中向服务器发送HTTP请求并获取响应。使用httpRequest可以实现异步数据交换和局部页面刷新,从而提高Web应用程序的性能和用户体验。
httpRequest包括以下几个步骤:
1. 创建XMLHttpRequest对象
2. 设置请求的方法、URL和是否异步
3. 发送请求
4. 监听XMLHttpRequest对象的状态变化和响应数据的到达
5. 处理响应数据
下面是一个简单的httpRequest示例:
```
function httpRequest(method, url, data, callback) {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
callback(xhr.responseText);
}
};
xhr.open(method, url, true);
xhr.setRequestHeader("Content-type", "application/json");
xhr.send(data);
}
httpRequest("POST", "/api/login", JSON.stringify({username: "test", password: "123456"}), function(response) {
console.log(response);
});
```
这个示例中,httpRequest函数接受四个参数:请求方法、请求URL、请求数据和响应回调函数。在函数内部,首先创建了一个XMLHttpRequest对象,然后设置了onreadystatechange事件处理函数,当XMLHttpRequest对象的状态变化时会触发该函数。在发送请求前设置了请求头,使用send方法发送请求,并将请求数据以JSON字符串的形式发送。最后,在响应回调函数中处理响应数据。
阅读全文
相关推荐
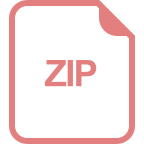
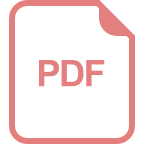
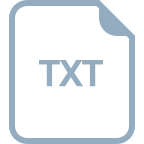













