使用springboot为框架的在线教育平台中,老师和学生用户如何一一对应?代码如何实现?
时间: 2024-02-23 14:00:53 浏览: 18
在Spring Boot中,我们可以使用Spring Security来实现用户认证和授权。对于在线教育平台中老师和学生的身份认证,我们可以通过自定义UserDetails和UserDetailsService来实现。
首先,我们需要定义一个包含用户信息的UserDetails类,例如:
```java
public class User implements UserDetails {
private String username; // 用户名
private String password; // 密码
private List<GrantedAuthority> authorities; // 用户权限列表
// 省略getter和setter方法
// ...
@Override
public Collection<? extends GrantedAuthority> getAuthorities() {
return this.authorities;
}
@Override
public String getUsername() {
return this.username;
}
@Override
public String getPassword() {
return this.password;
}
// 省略其他方法
// ...
}
```
然后,我们需要定义一个UserDetailsService接口的实现类,例如:
```java
@Service
public class UserDetailsServiceImpl implements UserDetailsService {
@Autowired
private UserService userService;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userService.getUserByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found");
}
List<GrantedAuthority> authorities = new ArrayList<>();
authorities.add(new SimpleGrantedAuthority(user.getRole()));
return new org.springframework.security.core.userdetails.User(user.getUsername(), user.getPassword(), authorities);
}
}
```
在上面的代码中,我们通过调用UserService的方法来获取对应用户名的用户信息,然后创建一个包含用户信息和权限信息的UserDetails对象。
最后,我们需要在Spring Security的配置中设置UserDetailsService,例如:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(passwordEncoder());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/teacher/**").hasRole("TEACHER")
.antMatchers("/student/**").hasRole("STUDENT")
.anyRequest().authenticated()
.and().formLogin().loginPage("/login").permitAll()
.and().logout().permitAll();
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
在上面的代码中,我们通过调用userDetailsService方法设置UserDetailsService,通过调用passwordEncoder方法设置密码加密方式,在configure(HttpSecurity http)方法中设置各个角色的访问权限。这样,当用户访问需要权限的资源时,Spring Security会自动调用UserDetailsService的loadUserByUsername方法进行身份认证,如果认证成功,则根据用户的角色信息进行授权。
需要注意的是,以上代码只是示例,具体实现需要根据具体的业务逻辑进行调整。
相关推荐
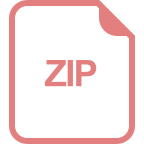
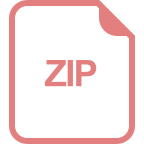
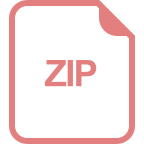














