如何使用python实现对DM二维码的矫正,并计算其中心坐标,偏移角度和二维码内容的0-1矩阵
时间: 2024-05-06 10:19:34 浏览: 9
要实现对DM二维码的矫正,需要进行以下步骤:
1. 导入相关库
```python
import cv2
import numpy as np
```
2. 读取二维码图片
```python
img = cv2.imread('qrcode.png')
```
3. 转换为灰度图像
```python
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
4. 进行二值化处理
```python
_, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)
```
5. 查找轮廓
```python
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
```
6. 找到最大的轮廓
```python
max_contour = max(contours, key=cv2.contourArea)
```
7. 进行透视变换
```python
rect = cv2.minAreaRect(max_contour)
box = np.int0(cv2.boxPoints(rect))
dst_pts = np.array([[0, 0], [0, 511], [511, 511], [511, 0]], dtype=np.float32)
M = cv2.getPerspectiveTransform(box, dst_pts)
dst = cv2.warpPerspective(img, M, (512, 512))
```
8. 计算其中心坐标和偏移角度
```python
center = rect[0]
angle = rect[2]
```
9. 提取二维码内容的0-1矩阵
```python
gray = cv2.cvtColor(dst, cv2.COLOR_BGR2GRAY)
_, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)
rows, cols = thresh.shape
matrix = np.zeros((rows // 8, cols // 8), dtype=np.int8)
for i in range(rows // 8):
for j in range(cols // 8):
matrix[i, j] = np.mean(thresh[i * 8:i * 8 + 8, j * 8:j * 8 + 8]) / 255
```
完整代码如下:
```python
import cv2
import numpy as np
img = cv2.imread('qrcode.png')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
_, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
max_contour = max(contours, key=cv2.contourArea)
rect = cv2.minAreaRect(max_contour)
box = np.int0(cv2.boxPoints(rect))
dst_pts = np.array([[0, 0], [0, 511], [511, 511], [511, 0]], dtype=np.float32)
M = cv2.getPerspectiveTransform(box, dst_pts)
dst = cv2.warpPerspective(img, M, (512, 512))
center = rect[0]
angle = rect[2]
gray = cv2.cvtColor(dst, cv2.COLOR_BGR2GRAY)
_, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)
rows, cols = thresh.shape
matrix = np.zeros((rows // 8, cols // 8), dtype=np.int8)
for i in range(rows // 8):
for j in range(cols // 8):
matrix[i, j] = np.mean(thresh[i * 8:i * 8 + 8, j * 8:j * 8 + 8]) / 255
print(center)
print(angle)
print(matrix)
```
相关推荐
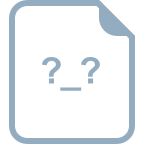
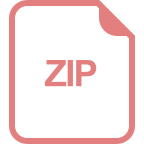














