JAVA线程实现下载_JAVA代码实现多线程下载
时间: 2023-12-06 13:02:05 浏览: 85
好的,我可以为您提供一段Java代码实现多线程下载:
```java
import java.io.InputStream;
import java.io.RandomAccessFile;
import java.net.HttpURLConnection;
import java.net.URL;
public class MultiThreadDownload extends Thread {
private String url; // 文件下载地址
private String filePath; // 保存路径
private int threadNum; // 线程数量
public MultiThreadDownload(String url, String filePath, int threadNum) {
this.url = url;
this.filePath = filePath;
this.threadNum = threadNum;
}
@Override
public void run() {
try {
URL downloadUrl = new URL(url);
HttpURLConnection httpURLConnection = (HttpURLConnection) downloadUrl.openConnection();
httpURLConnection.setRequestMethod("GET");
httpURLConnection.setConnectTimeout(5000);
int fileLength = httpURLConnection.getContentLength();
int blockSize = fileLength % threadNum == 0 ? fileLength / threadNum : fileLength / threadNum + 1;
RandomAccessFile randomAccessFile = new RandomAccessFile(filePath, "rw");
randomAccessFile.setLength(fileLength);
randomAccessFile.close();
for (int i = 0; i < threadNum; i++) {
int startIndex = i * blockSize;
int endIndex = (i + 1) * blockSize - 1;
if (endIndex > fileLength) endIndex = fileLength;
new DownloadThread(url, filePath, startIndex, endIndex).start();
}
} catch (Exception e) {
e.printStackTrace();
}
}
private class DownloadThread extends Thread {
private String url;
private String filePath;
private int startIndex;
private int endIndex;
public DownloadThread(String url, String filePath, int startIndex, int endIndex) {
this.url = url;
this.filePath = filePath;
this.startIndex = startIndex;
this.endIndex = endIndex;
}
@Override
public void run() {
try {
RandomAccessFile randomAccessFile = new RandomAccessFile(filePath, "rw");
randomAccessFile.seek(startIndex);
URL downloadUrl = new URL(url);
HttpURLConnection httpURLConnection = (HttpURLConnection) downloadUrl.openConnection();
httpURLConnection.setRequestMethod("GET");
httpURLConnection.setConnectTimeout(5000);
httpURLConnection.setRequestProperty("Range", "bytes=" + startIndex + "-" + endIndex);
InputStream inputStream = httpURLConnection.getInputStream();
byte[] buffer = new byte[1024];
int length = -1;
while ((length = inputStream.read(buffer)) != -1) {
randomAccessFile.write(buffer, 0, length);
}
inputStream.close();
randomAccessFile.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
```
这段代码利用多线程实现文件下载,具体实现过程为:首先获取文件长度,然后根据线程数量计算每个线程需要下载的文件块大小,接着创建多个下载线程,每个下载线程负责下载自己对应的文件块。在下载时,通过设置请求头中的 `Range` 参数实现分块下载,最终将所有分块拼接起来得到完整的文件。
阅读全文
相关推荐
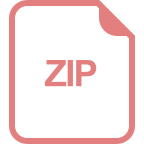
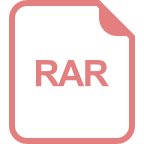
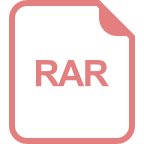
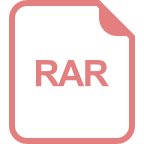
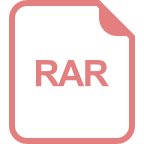
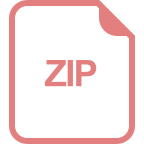
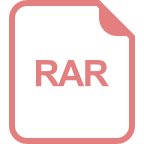
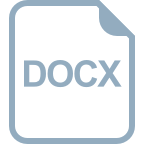
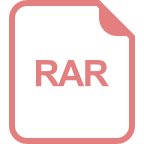
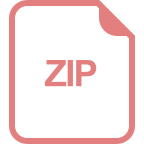
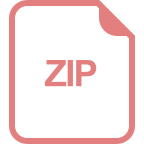
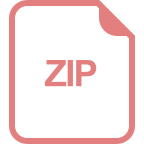
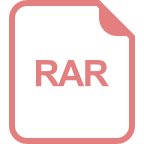
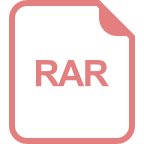
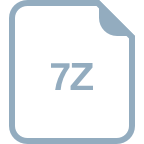