要求: 编写一个程序,可以在命令行输入参数,完成参数所指定的两幅真彩色位图图像的混合运算,并存储混合后的结果到新文件,命令行参数如下: blend x.bmp 80% y.bmp result.bmp 第1个参数( blend )为可执行程序名称; 第2个参数x.bmp为原始图像文件名; 第3个参数为混合比例(百分比),如80%表示x图像亮度数据 * 80% + y图像亮度数据* (1 - 80%) = 结果图像数据); 第4个参数y.bmp第二幅图像文件名; 第5个参数result.bmp为结果图像文件名。
时间: 2024-02-11 09:10:06 浏览: 144
好的,我明白了你的要求。以下是一个简单的 Python 程序,可以实现你所描述的功能:
```python
from PIL import Image
import sys
# 从命令行参数中获取文件名和混合比例
x_file = sys.argv[1]
blend_ratio = float(sys.argv[2].strip('%')) / 100
y_file = sys.argv[3]
result_file = sys.argv[4]
# 打开两幅图像文件
x = Image.open(x_file)
y = Image.open(y_file)
# 获取图像尺寸
width, height = x.size
# 创建新的图像对象
result = Image.new("RGB", (width, height))
# 进行混合运算
for x_coord in range(width):
for y_coord in range(height):
# 获取两幅图像的 RGB 值
x_rgb = x.getpixel((x_coord, y_coord))
y_rgb = y.getpixel((x_coord, y_coord))
# 计算混合后的 RGB 值
result_rgb = tuple(int(x * blend_ratio + y * (1 - blend_ratio)) for x, y in zip(x_rgb, y_rgb))
# 将新的 RGB 值写入结果图像
result.putpixel((x_coord, y_coord), result_rgb)
# 保存结果图像
result.save(result_file)
```
你可以在命令行中运行这个程序,例如:
```
python blend.py x.bmp 80% y.bmp result.bmp
```
这个程序会读取 x.bmp 和 y.bmp 两幅图像,并按照 80% 和 20% 的比例进行混合运算,最终将结果保存为 result.bmp。
阅读全文
相关推荐
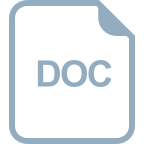
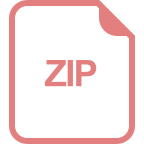
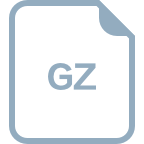


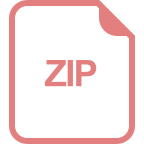
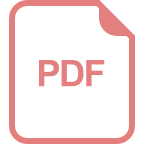
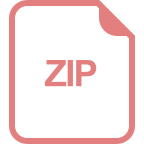
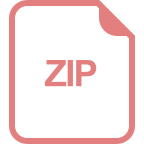
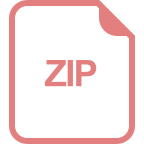
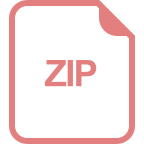
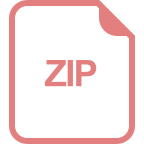
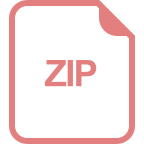
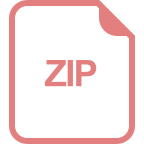
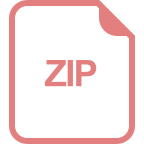
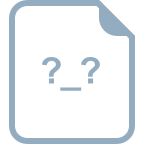
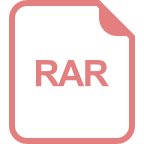
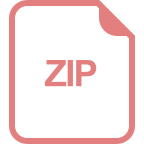