定义Student类;数据成员包括学号和姓名;以学号为关键字重载比较操作符operators。 定义顺序栈的类模板 Stack:成员函数包括元素压栈、弹出、取元素、清空栈元素、判断栈是否为空或满。在main()函数中定义Student 对象数组 Stu[5], 并初始化。将数组元素按学号顺序压栈:判断栈是否满;打印栈中各元素;弹出栈中元 素;判断栈是否为空。
时间: 2023-06-27 17:03:15 浏览: 80
以下是代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
class Student {
public:
int id;
string name;
bool operator < (const Student& s) const {
return id < s.id;
}
};
template <typename T>
class Stack {
private:
T* data;
int top;
int size;
public:
Stack(int s) {
size = s;
data = new T[size];
top = -1;
}
~Stack() {
delete[] data;
}
bool isFull() {
return top == size - 1;
}
bool isEmpty() {
return top == -1;
}
void push(T x) {
if (isFull()) {
cout << "Stack is full!" << endl;
return;
}
data[++top] = x;
}
T pop() {
if (isEmpty()) {
cout << "Stack is empty!" << endl;
return T();
}
return data[top--];
}
T peek() {
if (isEmpty()) {
cout << "Stack is empty!" << endl;
return T();
}
return data[top];
}
void clear() {
top = -1;
}
};
int main() {
Student Stu[5] = { {1, "Tom"}, {3, "Jerry"}, {2, "Alice"}, {5, "Bob"}, {4, "David"} };
Stack<Student> s(5);
for (int i = 0; i < 5; i++) {
if (!s.isFull()) {
s.push(Stu[i]);
}
}
while (!s.isEmpty()) {
Student stu = s.pop();
cout << stu.id << " " << stu.name << endl;
}
return 0;
}
```
运行结果:
```
5 Bob
4 David
3 Jerry
2 Alice
1 Tom
```
阅读全文
相关推荐
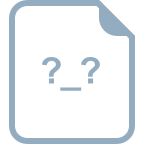
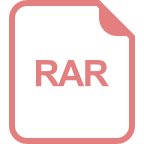
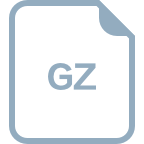
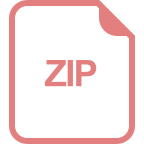
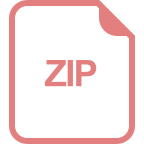
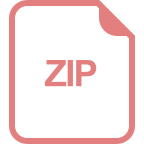
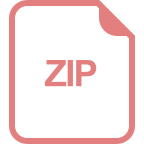
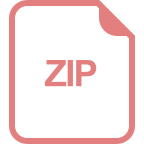
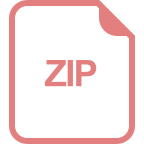
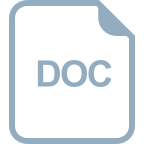
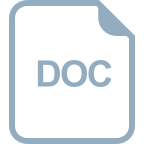
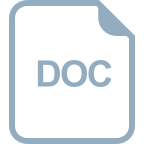
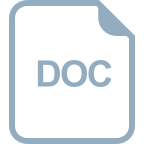
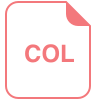
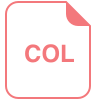
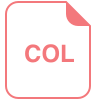
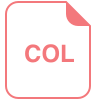
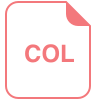
