#include <stdio.h> #include <stdlib.h> int main(int argc,char ** argv) { char *p; int i; p=malloc(30); strcpy(p,"not 30 bytes"); printf("p=<%s>\n",p); if(argc==2){ if(strcmp(argv[1],"-b")==0) p[50]='a'; else if(strcmp(argv[1],"-f")==0){ free(p); p[0]='b'; } } /*free(p);*/ return 0; }
时间: 2024-01-23 18:03:54 浏览: 145
这段代码也存在问题,尤其是最后的注释部分,如果取消注释的话,会导致程序崩溃或者出现其他未知的错误,因为p指针已经被释放掉了,但是在注释中又对其进行了释放操作。
如果要修正这个问题,可以在最后的注释部分中加入判断语句,判断p指针是否为NULL,如果不为NULL再进行释放操作。同时,建议将注释中的释放操作删除,保证程序的健壮性。
修改后的代码如下所示:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char** argv) {
char* p;
int i;
p = malloc(30);
strcpy(p, "not 30 bytes");
printf("p=<%s>\n", p);
if (argc == 2) {
if (strcmp(argv[1], "-b") == 0)
p[50] = 'a';
else if (strcmp(argv[1], "-f") == 0) {
free(p);
p = NULL;
}
}
if (p != NULL) {
free(p);
}
return 0;
}
```
在最后的判断语句中,加入了判断p指针是否为NULL的语句,如果不为NULL则进行释放操作。同时,注释中的释放操作也被删除了。
相关问题
#include <stdio.h> #include <stdlib.h> int main(int argc, char *argv[]) { printf("25600"); return 0; }
这段代码是一个简单的C语言程序,它的作用是输出数字25600。在程序中,使用了两个头文件<stdio.h>和<stdlib.h>,其中<stdio.h>包含了输入输出函数,而<stdlib.h>包含了一些常用的函数,例如atof()和atoi()。在main函数中,使用了三个参数,分别是argc、argv[]和return 0。其中,argc表示传递给程序的参数个数,argv[]表示传递给程序的参数列表,而return 0表示程序正常结束。在这个程序中,由于没有使用传递给程序的参数,因此argc和argv[]没有被使用到。
#define _CRT_SECURE_NO_WARNINGS #include<stdio.h> #include<string.h> #include<stdlib.h> int main(int argc, char* argv[]) { int a = 1; printf("\n%d\n", a % 2); system("pause"); return 0; }
你好!这是一个简单的C语言程序,它定义了一个宏 `_CRT_SECURE_NO_WARNINGS`,然后包含了一些头文件(stdio.h、string.h、stdlib.h)。在主函数中,声明了一个整型变量 a 并赋值为 1,然后使用 `%` 操作符计算 a 除以 2 的余数,并将结果打印出来。最后使用 `system("pause")` 函数来暂停程序的执行,以便查看输出结果。整个程序的返回值为 0,表示程序正常结束。
阅读全文
相关推荐
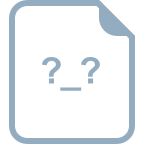
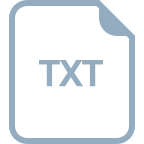







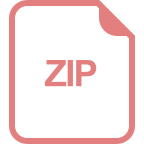
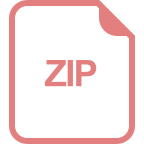
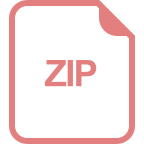
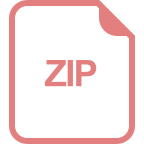
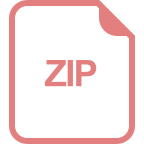