with torch.no_grad(): new_model.eval() features = new_model(img,datainput) 报错forward() takes 2 positional arguments but 3 were given, 如何修改代码
时间: 2024-01-16 09:03:01 浏览: 110
根据你提供的代码,可以看出 `img` 和 `datainput` 应该是两个不同的输入参数,但是在调用 `new_model` 的时候,却只传了一个参数。这就导致了报错提示中的 `forward() takes 2 positional arguments but 3 were given`。
要解决这个问题,可以将 `img` 和 `datainput` 合并成一个输入参数,如下所示:
```
with torch.no_grad():
new_model.eval()
features = new_model(img, datainput)
```
这样就只需要传入两个参数,而不是三个了。当然,这也要求你修改 `new_model` 的定义,使其接受两个参数作为输入。
相关问题
运行class GuidedBackpropReLUModel: def __init__(self, model, use_cuda): self.model = model self.model.eval() self.cuda = use_cuda if self.cuda: self.model = model.cuda() for idx, module in self.model.features._modules.items(): if module.__class__.__name__ == 'ReLU': self.model.features._modules[idx] = GuidedBackpropReLU() def forward(self, input): return self.model(input) def forward_static(self, input): if self.cuda: output = self.forward(input.cuda()) else: output = self.forward(input) return output def __call__(self, input, index = None): output = self.forward_static(input) if index == None: index = np.argmax(output.cpu().data.numpy()) one_hot = np.zeros((1, output.size()[-1]), dtype = np.float32) one_hot[0][index] = 1 one_hot = Variable(torch.from_numpy(one_hot), requires_grad = True) if self.cuda: one_hot = torch.sum(one_hot.cuda() * output) else: one_hot = torch.sum(one_hot * output) one_hot.backward() output = input.grad.cpu().data.numpy() output = output[0,:,:,:] return output报错Legacy autograd function with non-static forward method is deprecated. Please use new-style autograd function with static forward method. 如何修改代码
这个错误提示是因为代码中使用了旧版本的autograd函数,需要使用新版本的autograd函数。可以使用`@staticmethod`修饰`GuidedBackpropReLU`类中的`forward`方法,将其转换为静态方法。修改后的代码如下:
```
class GuidedBackpropReLU(nn.ReLU):
@staticmethod
def forward(input):
output = torch.clamp(input, min=0.0)
grad = torch.zeros_like(output)
grad[output > 0] = 1.0
output = output * grad
return output
class GuidedBackpropReLUModel:
def __init__(self, model, use_cuda):
self.model = model
self.model.eval()
self.cuda = use_cuda
if self.cuda:
self.model = model.cuda()
for idx, module in self.model.features._modules.items():
if module.__class__.__name__ == 'ReLU':
self.model.features._modules[idx] = GuidedBackpropReLU()
def forward(self, input):
return self.model(input)
def forward_static(self, input):
if self.cuda:
output = self.forward(input.cuda())
else:
output = self.forward(input)
return output
def __call__(self, input, index=None):
output = self.forward_static(input)
if index is None:
index = np.argmax(output.cpu().data.numpy())
one_hot = np.zeros((1, output.size()[-1]), dtype=np.float32)
one_hot[0][index] = 1
one_hot = Variable(torch.from_numpy(one_hot), requires_grad=True)
if self.cuda:
one_hot = torch.sum(one_hot.cuda() * output)
else:
one_hot = torch.sum(one_hot * output)
one_hot.backward()
output = input.grad.cpu().data.numpy()
output = output[0, :, :, :]
return output
```
features = new_model(img,datainput) 报错forward() takes 2 positional arguments but 3 were given, 应该如何修改
根据报错信息提示,`forward()` 方法只接受两个位置参数,但是在调用 `new_model` 的时候传入了三个参数,因此需要修改代码。
具体而言,可以将 `img` 和 `datainput` 合并成一个输入参数,然后将其作为一个参数传递给 `new_model`。具体代码如下:
```
with torch.no_grad():
new_model.eval()
features = new_model(img, datainput)
```
在这个代码中,我们将 `img` 和 `datainput` 合并成一个输入参数,并将其作为一个参数传递给 `new_model` 的 `forward()` 方法中。这样就可以避免报错了。
阅读全文
相关推荐
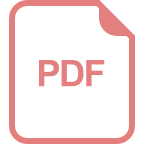
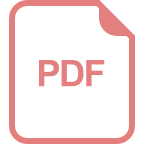
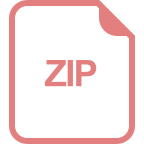
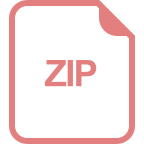
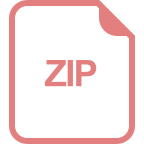
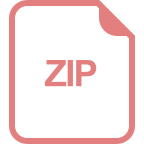
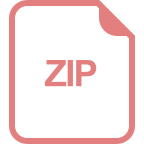
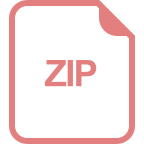
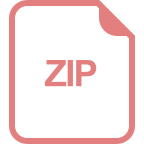
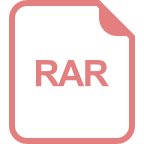
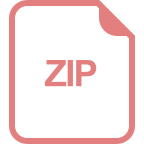
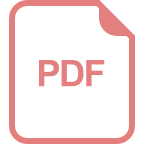
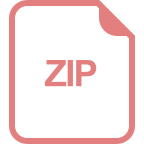
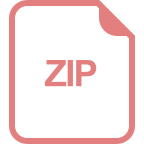
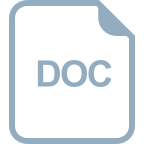