java怎么将数据库查询到的图片路径变成http返回给前台
时间: 2024-05-14 16:18:28 浏览: 82
在Java中将数据库查询到的图片路径变成http返回给前台,可以通过以下步骤实现:
1. 查询数据库获取图片路径。
2. 判断路径是否为空或者不存在,如果为空或者不存在,则返回空或者错误提示。
3. 如果路径存在,可以通过Java IO流将图片读取到内存中。
4. 将读取到的图片以字节数组的形式存储。
5. 将字节数组使用Base64编码。
6. 将编码后的字符串拼接成http请求返回给前台。
下面是一个简单的Java代码示例:
```java
@RequestMapping("/getImage")
@ResponseBody
public String getImage(@RequestParam("id") String id, HttpServletResponse response) {
// 查询数据库获取图片路径
String imagePath = getImagePathFromDB(id);
if (imagePath == null || imagePath.length() == 0) {
return "error";
}
// 读取图片
File imageFile = new File(imagePath);
if (!imageFile.exists()) {
return "error";
}
byte[] imageData = null;
try {
InputStream is = new FileInputStream(imageFile);
imageData = new byte[is.available()];
is.read(imageData);
is.close();
} catch (IOException e) {
e.printStackTrace();
return "error";
}
// Base64编码
String base64Image = Base64.getEncoder().encodeToString(imageData);
// 拼接http请求返回给前台
StringBuilder sb = new StringBuilder();
sb.append("data:image/png;base64,");
sb.append(base64Image);
return sb.toString();
}
```
在上面的代码中,我们通过`@RequestParam`注解获取了前台传入的`id`参数,然后调用`getImagePathFromDB`方法从数据库中查询图片路径。如果图片路径为空或者不存在,我们返回了`error`字符串。如果图片路径存在,我们使用Java IO流将图片读取到内存中,然后使用Base64编码将其转换成字符串,最后拼接成http请求返回给前台。
阅读全文
相关推荐
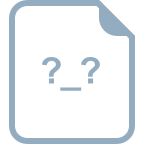
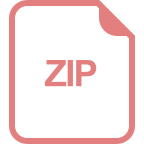
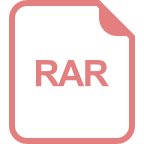
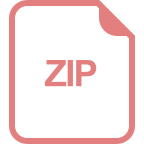
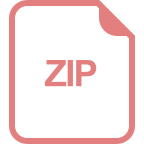
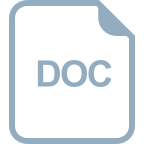
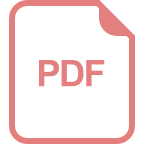
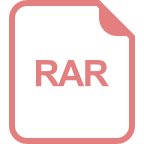
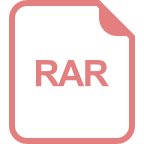
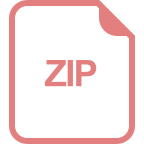
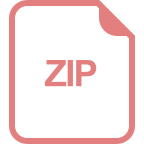
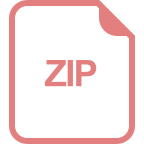
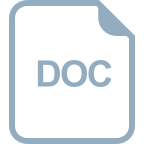
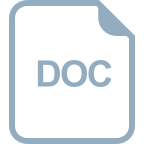
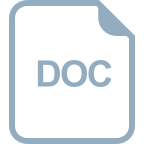
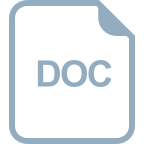
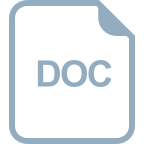
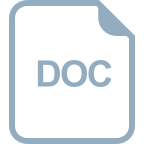
